The Sed object¶
This example demonstrates the various methods associated with the Sed
class.
Sed
objects can be extracted directly from Grid objects or created by Galaxy objects.
We start by loading some modules and initialising a grid:
[1]:
import matplotlib.pyplot as plt
import numpy as np
from unyt import Angstrom, Hz, erg, eV, s, um
from synthesizer.emission_models.attenuation.igm import Madau96
from synthesizer.filters import FilterCollection
from synthesizer.grid import Grid
from synthesizer.sed import Sed, plot_spectra_as_rainbow
grid_dir = "../../../tests/test_grid/"
grid_name = "test_grid"
grid = Grid(grid_name, grid_dir=grid_dir)
Next, let’s extract the spectra at a given point in our grid. We first define a target age and metallicity, obtain the index of the grid at these values, then extract the spectra. This will be in the form of an Sed
object.
[2]:
log10age = 6.0 # log10(age/yr)
metallicity = 0.01
spectra_id = "incident"
grid_point = grid.get_grid_point(log10ages=log10age, metallicity=metallicity)
sed = grid.get_spectra(grid_point, spectra_id=spectra_id)
sed.lnu *= 1e8 # multiply initial stellar mass
print(sed)
+-------------------------------------------------------------------------------------------------+
| SED |
+---------------------------+---------------------------------------------------------------------+
| Attribute | Value |
+---------------------------+---------------------------------------------------------------------+
| redshift | 0 |
+---------------------------+---------------------------------------------------------------------+
| ndim | 1 |
+---------------------------+---------------------------------------------------------------------+
| shape | (9244,) |
+---------------------------+---------------------------------------------------------------------+
| lam (9244,) | 1.30e-04 Å -> 2.99e+11 Å (Mean: 9.73e+09 Å) |
+---------------------------+---------------------------------------------------------------------+
| nu (9244,) | 1.00e+07 Hz -> 2.31e+22 Hz (Mean: 8.51e+19 Hz) |
+---------------------------+---------------------------------------------------------------------+
| lnu (9244,) | 0.00e+00 erg/(Hz*s) -> 3.10e+29 erg/(Hz*s) (Mean: 9.16e+27 erg) |
+---------------------------+---------------------------------------------------------------------+
| bolometric_luminosity | 7.016148283811726e+44 erg/s |
+---------------------------+---------------------------------------------------------------------+
| bolometric_luminosity | 7.016148283811726e+44 erg/s |
+---------------------------+---------------------------------------------------------------------+
| energy (9244,) | 4.14e-08 eV -> 9.56e+07 eV (Mean: 3.52e+05 eV) |
+---------------------------+---------------------------------------------------------------------+
| frequency (9244,) | 1.00e+07 Hz -> 2.31e+22 Hz (Mean: 8.51e+19 Hz) |
+---------------------------+---------------------------------------------------------------------+
| llam (9244,) | 0.00e+00 erg/(s*Å) -> 1.27e+42 erg/(s*Å) (Mean: 2.79e+40 erg/(s*Å)) |
+---------------------------+---------------------------------------------------------------------+
| luminosity (9244,) | 0.00e+00 erg/s -> 9.95e+44 erg/s (Mean: 2.28e+43 erg/s) |
+---------------------------+---------------------------------------------------------------------+
| luminosity_lambda (9244,) | 0.00e+00 erg/(s*Å) -> 1.27e+42 erg/(s*Å) (Mean: 2.79e+40 erg/(s*Å)) |
+---------------------------+---------------------------------------------------------------------+
| luminosity_nu (9244,) | 0.00e+00 erg/(Hz*s) -> 3.10e+29 erg/(Hz*s) (Mean: 9.16e+27 erg) |
+---------------------------+---------------------------------------------------------------------+
| wavelength (9244,) | 1.30e-04 Å -> 2.99e+11 Å (Mean: 9.73e+09 Å) |
+---------------------------+---------------------------------------------------------------------+
Sed
objects contain a wavelength grid and luminosity in the lam
and lnu
attributes. Both come with units making them easy to convert:
[3]:
print(sed.lam)
print(sed.lnu)
[1.29662e-04 1.33601e-04 1.37660e-04 ... 2.97304e+11 2.98297e+11
2.99293e+11] Å
[0. 0. 0. ... 0. 0. 0.] erg/(Hz*s)
These also have more descriptive aliases:
[4]:
print(sed.wavelength)
print(sed.luminosity_nu)
[1.29662e-04 1.33601e-04 1.37660e-04 ... 2.97304e+11 2.98297e+11
2.99293e+11] Å
[0. 0. 0. ... 0. 0. 0.] erg/(Hz*s)
Thus we can easily make a plot:
[5]:
plt.plot(np.log10(sed.lam), np.log10(sed.lnu))
plt.show()
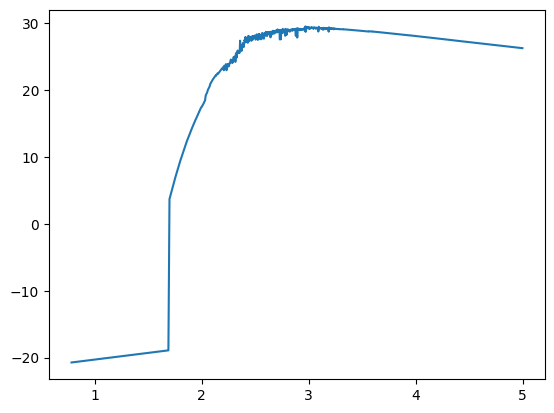
We can also also visualise the spectrum as a rainbow.
[6]:
fig, ax = plot_spectra_as_rainbow(sed)
plt.show()
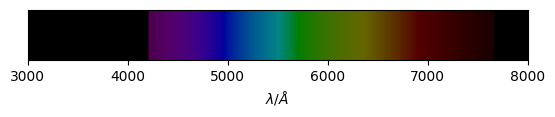
We can also get the luminosity (\(L\)) or spectral luminosity density per \(\AA\) (\(L_{\lambda}\)):
[7]:
print(sed.luminosity)
print(sed.llam)
[0. 0. 0. ... 0. 0. 0.] erg/s
[0. 0. 0. ... 0. 0. 0.] erg/(s*Å)
Sed
objects can be easily scaled via the *
operator. For example,
[8]:
sed_x_10 = sed * 10
plt.plot(np.log10(sed.lam), np.log10(sed.lnu))
plt.plot(np.log10(sed_x_10.lam), np.log10(sed_x_10.lnu))
plt.show()
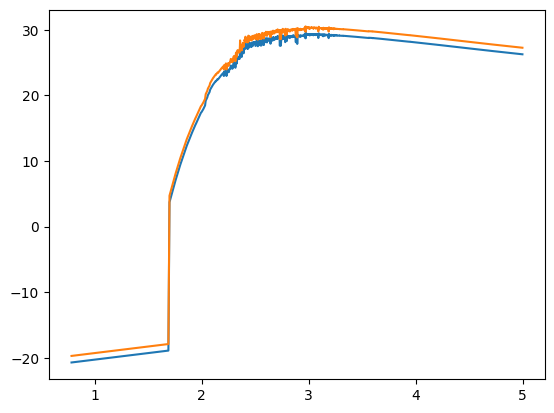
Methods¶
There are a number of helper methods on Sed
for calculating common derived properties. We provide some examples below.
We can calculate the integrated bolometric luminosity of the Sed using the bolometric_luminosity
property method:
[9]:
sed.bolometric_luminosity
[9]:
unyt_quantity(7.01614828e+44, 'erg/s')
By default any spectra integration will use a trapezoid method. It’s also possible to use the simpson rule using the measure_bolometric_luminosity
method.
[10]:
sed.measure_bolometric_luminosity(integration_method="simps")
[10]:
unyt_quantity(6.99398441e+44, 'erg/s')
You can also get the luminosity or lnu
in a particular window by passing the wavelengths defining the limits of the window.
[11]:
sed.measure_window_luminosity((1400.0 * Angstrom, 1600.0 * Angstrom))
[11]:
unyt_quantity(4.0480619e+43, 'erg/s')
Notice how units were provided with this window. These are required but also allow you to use an arbitrary unit system.
[12]:
sed.measure_window_luminosity((0.14 * um, 0.16 * um))
[12]:
unyt_quantity(4.0480619e+43, 'erg/s')
[13]:
sed.measure_window_lnu((1400.0 * Angstrom, 1600.0 * Angstrom))
[13]:
unyt_quantity(1.51428832e+29, 'erg/(Hz*s)')
As for the bolometric luminosity, there are multiple integration methods that can be used for calculating the window. By default synthesizer will use the trapezoid method ("trapz"
), but you can also take a simple average.
[14]:
sed.measure_window_lnu(
(1400.0 * Angstrom, 1600.0 * Angstrom), integration_method="average"
)
[14]:
unyt_quantity(1.5142835e+29, 'erg/(Hz*s)')
We can measure a spectral break by providing two windows:
[15]:
sed.measure_break((3400, 3600) * Angstrom, (4150, 4250) * Angstrom)
[15]:
np.float64(0.8556966386986629)
There are also a few in-built break methods, e.g. measure_Balmer_break()
[16]:
sed.measure_balmer_break()
[16]:
np.float64(0.8556966386986629)
[17]:
sed.measure_d4000()
[17]:
np.float64(0.9014335946523936)
We can also measure absorption line indices, and spectral slopes (e.g. the UV spectral slope \(\beta\)):
[18]:
sed.measure_index(
(1500, 1600) * Angstrom, (1400, 1500) * Angstrom, (1600, 1700) * Angstrom
)
[18]:
unyt_quantity(5.80884398, 'Å')
[19]:
sed.measure_beta()
[19]:
np.float64(-2.9472901626578625)
By default this uses a single window and fits the spectrum by a power-law. However, we can also specify two windows as below, in which case the luminosity in each window is calcualted and used to infer a slope:
[20]:
sed.measure_beta(window=(1250, 1750, 2250, 2750) * Angstrom)
[20]:
np.float64(-2.9533621846623603)
Finally, we can also measure ionising photon production rates at a particular ionisation energy:
[21]:
sed.calculate_ionising_photon_production_rate(
ionisation_energy=13.6 * eV, limit=1000
)
[21]:
unyt_quantity(9.82125014e+54, '1/s')
Observed frame SED¶
By default Sed
objects are defined in the rest frame. To move an SED to the observer frame we need to provide a cosmology, using an astropy.cosmology
object, a redshift \(z\), and optionally an IGM absorption model (see here for details).
[22]:
from astropy.cosmology import Planck18 as cosmo
z = 3.0 # redshift
sed.get_fnu(cosmo, z, igm=Madau96) # generate observed frame spectra
plt.plot(np.log10(sed.obslam), np.log10(sed.fnu))
plt.show()
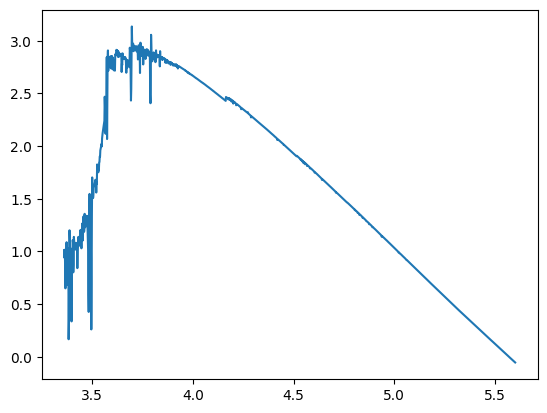
Photometry¶
Once we have computed the observed frame SED there is a method on an Sed
object that allows us to calculate observed photometry (the same is of course true for rest frame photometry). However, first we need to instantiate a FilterCollection
object (see Filters for details):
[23]:
filter_codes = [
f"JWST/NIRCam.{f}"
for f in [
"F070W",
"F090W",
"F115W",
"F150W",
"F200W",
"F277W",
"F356W",
"F444W",
]
] # define a list of filter codes
fc = FilterCollection(filter_codes, new_lam=grid.lam)
# Measure observed photometry
fluxes = sed.get_photo_fnu(fc)
print(fluxes)
------------------------------------------------------------------
| PHOTOMETRY (FLUX) |
|------------------------------------|---------------------------|
| JWST/NIRCam.F070W (λ = 7.04e+03 Å) | 6.76e-30 erg/(Hz*cm**2*s) |
|------------------------------------|---------------------------|
| JWST/NIRCam.F090W (λ = 9.02e+03 Å) | 5.34e-30 erg/(Hz*cm**2*s) |
|------------------------------------|---------------------------|
| JWST/NIRCam.F115W (λ = 1.15e+04 Å) | 3.86e-30 erg/(Hz*cm**2*s) |
|------------------------------------|---------------------------|
| JWST/NIRCam.F150W (λ = 1.50e+04 Å) | 2.77e-30 erg/(Hz*cm**2*s) |
|------------------------------------|---------------------------|
| JWST/NIRCam.F200W (λ = 1.99e+04 Å) | 1.90e-30 erg/(Hz*cm**2*s) |
|------------------------------------|---------------------------|
| JWST/NIRCam.F277W (λ = 2.76e+04 Å) | 1.10e-30 erg/(Hz*cm**2*s) |
|------------------------------------|---------------------------|
| JWST/NIRCam.F356W (λ = 3.57e+04 Å) | 7.10e-31 erg/(Hz*cm**2*s) |
|------------------------------------|---------------------------|
| JWST/NIRCam.F444W (λ = 4.40e+04 Å) | 4.93e-31 erg/(Hz*cm**2*s) |
------------------------------------------------------------------
Multiple SEDs¶
An Sed
object can be composed of multiple SEDs ,if their dimensions are identical (i.e. same wavelength resolution). This is common when we are, for example, modelling the spectra of particle based galaxy.
To demonstrate, let’s create an Sed
object with two seds:
[24]:
sed2 = Sed(sed.lam, np.array([sed.lnu, sed.lnu * 2]) * erg / s / Hz)
print(sed2.lnu.shape)
print(sed2.measure_window_lnu((1400, 1600) * Angstrom))
print(sed2.measure_beta())
print(sed2.measure_beta(window=(1250, 1750, 2250, 2750) * Angstrom))
print(sed2.measure_balmer_break())
print(
sed2.measure_index(
(1500, 1600) * Angstrom,
(1400, 1500) * Angstrom,
(1600, 1700) * Angstrom,
)
)
print(
sed2.calculate_ionising_photon_production_rate(
ionisation_energy=13.6 * eV, limit=1000
)
)
(2, 9244)
[1.51428832e+29 3.02857663e+29] erg/(Hz*s)
[-2.94729016 -2.94729016]
[-2.95336218 -2.95336218]
[0.85569664 0.85569664]
[5.80884398 5.80884398] Å
[9.82125014e+54 1.96425003e+55] 1/s
This even works for N-dimensional spectra. For example, if we convert an entire grid into a set of spectra:
[25]:
sed3 = grid.get_sed(spectra_type="incident")
print(sed3.lnu.shape)
print(sed3.measure_window_lnu((1400, 1600) * Angstrom))
print(
sed3.calculate_ionising_photon_production_rate(
ionisation_energy=13.6 * eV, limit=1000
)
)
(51, 13, 9244)
[[1.13471342e+21 1.14549400e+21 1.19827652e+21 1.21735974e+21
1.23427750e+21 1.25229181e+21 1.32870600e+21 1.38141712e+21
1.51428832e+21 1.54411152e+21 1.70919609e+21 1.99890603e+21
2.08142719e+21]
[1.23658203e+21 1.23480644e+21 1.31621049e+21 1.35910523e+21
1.41968475e+21 1.49306939e+21 1.62206995e+21 1.69922787e+21
1.88457815e+21 1.79482547e+21 1.99235637e+21 2.14295067e+21
2.06529441e+21]
[1.27968613e+21 1.28268928e+21 1.43858757e+21 1.55324407e+21
1.66373822e+21 1.78864595e+21 1.87670183e+21 1.94432408e+21
2.08871139e+21 1.86010327e+21 2.01554736e+21 2.03687890e+21
1.89816381e+21]
[1.37472829e+21 1.44716139e+21 1.75673573e+21 1.88740194e+21
1.97785166e+21 2.09475896e+21 2.18451223e+21 2.15968500e+21
2.22536681e+21 1.81417151e+21 1.93594989e+21 1.85543289e+21
1.77597286e+21]
[1.43977772e+21 1.57558510e+21 1.68780234e+21 1.80023072e+21
1.85320415e+21 1.91363055e+21 1.97327293e+21 1.91543965e+21
1.94875514e+21 1.78229174e+21 1.68836056e+21 1.62288574e+21
1.56413896e+21]
[1.14971395e+21 1.23902487e+21 1.33894960e+21 1.36757732e+21
1.37623441e+21 1.39434569e+21 1.43402067e+21 1.42919712e+21
1.48416276e+21 1.44644557e+21 1.36542548e+21 1.31502497e+21
1.23235699e+21]
[9.48488723e+20 1.00033035e+21 1.04556602e+21 1.05414003e+21
1.04963162e+21 1.05408471e+21 1.07983455e+21 1.05459894e+21
1.08798171e+21 1.03549423e+21 9.75603629e+20 9.06490762e+20
8.39284041e+20]
[7.71694854e+20 7.94344071e+20 7.92855934e+20 8.08451212e+20
8.05243983e+20 8.03520703e+20 8.14072749e+20 7.92301022e+20
8.10833642e+20 7.62224362e+20 7.10525423e+20 6.40590197e+20
5.76224403e+20]
[6.07103184e+20 6.28215666e+20 5.77841632e+20 5.82527557e+20
5.83413332e+20 5.95029874e+20 5.85098842e+20 5.67781981e+20
5.79033158e+20 5.45552100e+20 5.03425001e+20 4.46646939e+20
4.12221982e+20]
[4.76653582e+20 4.97188760e+20 4.47895977e+20 4.39033127e+20
4.30731139e+20 4.34903241e+20 4.26861835e+20 4.10257217e+20
4.09754084e+20 3.85421743e+20 3.45257550e+20 3.02841750e+20
2.70198604e+20]
[3.63660740e+20 3.73347632e+20 3.32675208e+20 3.19073738e+20
3.04328400e+20 2.97856832e+20 2.88945097e+20 2.76465128e+20
2.77281748e+20 2.60247383e+20 2.33548601e+20 2.05938683e+20
1.85235698e+20]
[2.72750628e+20 2.77250196e+20 2.47813520e+20 2.28578607e+20
2.13988596e+20 2.08464458e+20 2.05243991e+20 1.93795245e+20
1.91494347e+20 1.78270795e+20 1.58778606e+20 1.43468433e+20
1.29898760e+20]
[1.97658391e+20 2.02508727e+20 1.81221213e+20 1.65981422e+20
1.54442924e+20 1.49720789e+20 1.44712300e+20 1.39886052e+20
1.36816074e+20 1.26540999e+20 1.14838394e+20 1.03484590e+20
9.27111225e+19]
[1.54612491e+20 1.53414741e+20 1.31679933e+20 1.23848658e+20
1.16710284e+20 1.12490060e+20 1.08353761e+20 1.02233617e+20
9.99148730e+19 9.25457794e+19 8.48100652e+19 7.60543865e+19
6.98599432e+19]
[1.17121123e+20 1.13510715e+20 1.00717257e+20 9.40045718e+19
9.06210027e+19 8.82135591e+19 8.48968890e+19 8.12701681e+19
7.74480879e+19 7.32138756e+19 6.76927334e+19 5.92813895e+19
5.49158605e+19]
[9.09868331e+19 8.96467286e+19 7.93089724e+19 7.32361433e+19
7.02594643e+19 6.84881408e+19 6.55990341e+19 6.41648169e+19
6.05305711e+19 5.70334869e+19 5.25070367e+19 4.67194497e+19
4.33644349e+19]
[7.14946326e+19 7.29864137e+19 6.20503850e+19 5.67246532e+19
5.45553140e+19 5.26774514e+19 5.13171211e+19 4.96005096e+19
4.72763185e+19 4.42085873e+19 4.11321823e+19 3.62706353e+19
3.35773496e+19]
[5.77183198e+19 5.76807480e+19 4.79363468e+19 4.41261927e+19
4.32362825e+19 4.17316879e+19 4.07713357e+19 3.92290156e+19
3.68412888e+19 3.48941220e+19 3.16864035e+19 2.73268655e+19
2.46412634e+19]
[4.60027101e+19 4.39831569e+19 3.84790152e+19 3.57897208e+19
3.39662891e+19 3.26626159e+19 3.14167891e+19 3.02769153e+19
2.77600160e+19 2.63395618e+19 2.40697808e+19 2.04268096e+19
1.84598475e+19]
[3.92072895e+19 3.65967885e+19 3.10220956e+19 2.90992218e+19
2.75122426e+19 2.67847610e+19 2.48941815e+19 2.37551675e+19
2.16869101e+19 1.99331720e+19 1.79271661e+19 1.50851564e+19
1.35262900e+19]
[2.91061786e+19 2.83431774e+19 2.40118555e+19 2.25693887e+19
2.20661992e+19 2.10925852e+19 1.95844381e+19 1.82528466e+19
1.68352142e+19 1.54154659e+19 1.34305410e+19 1.11804995e+19
9.41507583e+18]
[2.36915872e+19 2.12768366e+19 1.81941116e+19 1.71145928e+19
1.66590944e+19 1.58445011e+19 1.46070051e+19 1.36194374e+19
1.19053267e+19 1.08620176e+19 9.46783949e+18 7.77239264e+18
6.36089546e+18]
[1.81636049e+19 1.62716647e+19 1.37410379e+19 1.32075629e+19
1.22013529e+19 1.21744297e+19 1.10500936e+19 9.99592972e+18
8.90324565e+18 7.75248365e+18 6.39221453e+18 4.97779559e+18
4.04612548e+18]
[1.35143376e+19 1.19089413e+19 9.96107269e+18 9.52009962e+18
8.50606510e+18 8.71240318e+18 7.94464186e+18 6.95346073e+18
5.94833435e+18 5.03452349e+18 3.97542231e+18 3.07010636e+18
2.28941164e+18]
[1.04066785e+19 9.52819787e+18 7.08292851e+18 6.62841785e+18
6.00193433e+18 5.71272264e+18 5.23550248e+18 4.56588917e+18
3.85114663e+18 3.14642423e+18 2.38040151e+18 1.63303194e+18
1.03956887e+18]
[8.07187060e+18 6.66754211e+18 4.61248037e+18 4.26753927e+18
3.95447496e+18 3.74659580e+18 3.34364430e+18 2.86821932e+18
2.44913428e+18 1.73049072e+18 1.16502431e+18 6.46787115e+17
4.10059717e+17]
[5.71828668e+18 4.70060871e+18 3.26416225e+18 3.03293714e+18
2.57241787e+18 2.45168364e+18 2.05039573e+18 1.64639765e+18
1.19824030e+18 8.20075029e+17 5.58288896e+17 2.55107609e+17
2.14928670e+17]
[3.91697861e+18 3.10898077e+18 2.23193261e+18 1.88212584e+18
1.60181065e+18 1.42418417e+18 1.07155968e+18 8.00261464e+17
5.45633606e+17 3.60814789e+17 2.51257561e+17 1.51728809e+17
1.07468201e+17]
[3.07207565e+18 2.21490002e+18 1.59985002e+18 1.27289808e+18
9.86635376e+17 7.89017816e+17 5.02532889e+17 3.12304697e+17
2.47266697e+17 1.91841510e+17 1.34201801e+17 8.07736145e+16
5.13315113e+16]
[2.21412429e+18 1.70739698e+18 1.12456299e+18 8.08991943e+17
5.49339039e+17 3.87645752e+17 2.05796362e+17 1.13104733e+17
9.58521771e+16 8.27892437e+16 5.82433613e+16 4.89395647e+16
3.10766840e+16]
[1.83999174e+18 1.21884117e+18 7.51630527e+17 4.71711372e+17
2.98598222e+17 1.78368899e+17 8.35243686e+16 5.51498774e+16
3.30124426e+16 2.55179336e+16 3.07254291e+16 2.40304782e+16
1.95892429e+16]
[1.15531869e+18 8.06228667e+17 4.12260754e+17 2.15071734e+17
1.25267443e+17 6.82891806e+16 4.76437098e+16 3.77408509e+16
2.19589554e+16 1.92825866e+16 1.49308978e+16 7.56505169e+15
8.33368855e+15]
[7.89781905e+17 4.96656817e+17 1.89864442e+17 7.74930492e+16
4.16664460e+16 3.81795368e+16 2.22582655e+16 1.81619482e+16
1.50786138e+16 1.48879163e+16 8.09134907e+15 4.68596797e+15
2.56578090e+15]
[4.72191322e+17 2.98275334e+17 6.28496032e+16 2.70647434e+16
2.09574794e+16 2.21249826e+16 1.43973094e+16 8.72762623e+15
8.92311891e+15 6.96512854e+15 5.26878970e+15 2.89596268e+15
2.30117140e+15]
[2.77452412e+17 1.66538805e+17 1.65232375e+16 1.35880269e+16
9.36888279e+15 5.89247599e+15 4.70342915e+15 5.53609197e+15
3.45596753e+15 2.54339332e+15 1.97815460e+15 1.60390905e+15
1.03603596e+15]
[1.53475171e+17 7.52302167e+16 6.92491285e+15 3.19641213e+15
3.14084521e+15 2.33249098e+15 2.28324281e+15 1.34152900e+15
1.45429770e+15 9.10395422e+14 8.73227711e+14 8.83868756e+14
7.53241256e+14]
[9.25232630e+16 2.90853647e+16 2.99937324e+15 1.28259868e+15
1.31303247e+15 9.68395408e+14 6.74377515e+14 7.42675011e+14
6.98759821e+14 3.95089661e+14 5.49557983e+14 5.14500193e+14
6.29753805e+14]
[5.46350858e+16 1.00715999e+16 9.24462641e+14 7.95903690e+14
6.03468919e+14 5.34110735e+14 2.52686784e+14 4.59259242e+14
4.58261251e+14 3.92692714e+14 4.22339519e+14 4.24654761e+14
3.14236176e+14]
[3.60039387e+16 1.19786189e+16 1.02063163e+15 8.45221800e+14
5.89463658e+14 2.12566906e+14 4.18529776e+14 3.81006568e+14
3.33768516e+14 3.34376351e+14 2.96860161e+14 2.15272250e+14
3.21711799e+14]
[3.72013664e+16 2.17753872e+16 5.96075410e+14 1.19610109e+14
3.15780591e+14 3.86914335e+14 3.78740847e+14 1.62611184e+14
1.82993540e+14 3.00789645e+14 2.37368175e+14 2.65058231e+14
1.26432126e+15]
[3.37520077e+16 2.86824893e+16 1.78999170e+14 4.42632665e+14
3.69849353e+14 3.33325859e+14 1.00709973e+14 2.58184023e+14
3.15787067e+14 9.97393128e+13 1.58344723e+14 2.22245895e+14
1.45458099e+16]
[3.61919242e+16 3.78797565e+16 8.49853724e+14 7.55009947e+13
1.11484434e+14 9.15045980e+13 3.00020673e+14 2.61492222e+14
9.24374717e+13 2.33713740e+14 1.71843242e+14 1.26369029e+14
7.66763195e+15]
[2.22397960e+16 2.45321742e+16 3.70916118e+15 4.20930011e+14
4.28265836e+14 3.68542340e+14 9.59929484e+13 9.02873077e+13
2.26779036e+14 1.79320270e+14 7.19460334e+13 7.82629903e+15
6.31018064e+15]
[1.93221625e+16 2.32514419e+16 1.50810779e+16 2.65088031e+14
8.26190889e+13 1.00406144e+14 2.74046976e+14 2.77355297e+14
2.39716488e+14 8.27069504e+13 1.17013965e+14 5.99210382e+15
4.59727585e+15]
[2.63409991e+16 2.22879873e+16 1.39819283e+16 4.36892945e+15
3.01797181e+13 5.32605507e+13 9.78228948e+13 8.26656778e+13
1.37562618e+14 2.27691230e+14 1.49304893e+15 4.64023034e+15
5.09123842e+15]
[1.69088714e+16 2.00950365e+16 1.58345473e+16 8.60724042e+15
1.11564906e+15 2.87287469e+13 3.13455634e+13 3.31300713e+13
3.18564857e+13 1.36124014e+14 1.21430355e+16 5.44516484e+15
1.98254259e+15]
[8.10817255e+15 1.65421091e+16 1.86664723e+16 1.10130439e+16
5.26891916e+15 2.63100528e+15 8.03199152e+14 5.01471952e+14
6.84937006e+13 4.40597564e+15 3.66309672e+15 2.75862939e+15
2.87387425e+15]
[1.21732117e+16 1.45695046e+16 7.69508269e+15 1.40881173e+16
8.23025391e+15 6.81318021e+15 5.03545587e+15 4.19445986e+15
3.53537613e+15 7.54163268e+15 2.94771226e+15 3.23585485e+15
3.37093497e+15]
[8.59649849e+15 7.89889243e+15 8.87188466e+15 4.60362143e+15
1.02582736e+16 3.95248060e+15 8.05515792e+15 7.88183465e+15
6.32624046e+15 2.97566517e+15 2.99688376e+15 1.41709912e+15
1.18147534e+15]
[4.20913977e+14 2.93866635e+15 5.56015649e+15 6.27577917e+15
5.49532087e+15 7.54855173e+15 2.88643111e+15 3.29035365e+15
1.36273058e+15 2.81932867e+15 1.16796003e+15 2.98134864e+15
1.09140605e+15]
[4.20494033e+13 2.35493321e+15 2.73807698e+11 2.22911949e+15
1.27438019e+13 1.70952081e+15 1.44902857e+15 1.41191896e+15
1.33719621e+15 1.17677821e+15 1.02554857e+15 1.73078483e+15
9.72714855e+14]] erg/(Hz*s)
[[9.74118552e+46 9.67247808e+46 9.80372121e+46 9.98162182e+46
1.01102135e+47 1.02761135e+47 1.03770575e+47 1.01203968e+47
9.82125014e+46 9.07532255e+46 8.30385395e+46 6.90139191e+46
6.01016385e+46]
[1.11420279e+47 1.10148840e+47 1.16376656e+47 1.16330315e+47
1.13119529e+47 1.11155199e+47 1.06471799e+47 9.93300080e+46
9.05423079e+46 8.13751020e+46 6.75209770e+46 5.21526138e+46
4.57805143e+46]
[1.20025506e+47 1.19718515e+47 1.21385057e+47 1.16197436e+47
1.09861672e+47 1.07627685e+47 9.98339656e+46 9.07536067e+46
8.01419170e+46 7.46444683e+46 5.85602875e+46 4.48181479e+46
3.87800288e+46]
[1.29731060e+47 1.26920029e+47 1.11904841e+47 1.03334313e+47
9.54284614e+46 8.84348427e+46 8.20565240e+46 7.26925509e+46
6.37452966e+46 6.20491360e+46 4.71281527e+46 3.45866440e+46
2.87114914e+46]
[1.18870830e+47 1.08225489e+47 9.74010226e+46 9.01196160e+46
8.06777050e+46 7.62673592e+46 6.85336700e+46 6.04918795e+46
5.14000782e+46 4.13817668e+46 3.58559483e+46 2.56410742e+46
2.09409837e+46]
[8.25397384e+46 7.32248110e+46 6.85831940e+46 6.52962637e+46
6.11018516e+46 5.69815158e+46 4.69896384e+46 4.19793037e+46
3.61498866e+46 2.89469107e+46 2.57460552e+46 1.86222512e+46
1.68102097e+46]
[5.74731542e+46 5.23072316e+46 4.38175143e+46 4.22299640e+46
3.91708971e+46 3.49284815e+46 2.84784548e+46 2.54602282e+46
2.19916493e+46 1.72025743e+46 1.51102297e+46 9.78093612e+45
7.90967793e+45]
[4.29813850e+46 3.74135258e+46 2.70121365e+46 2.34241023e+46
2.22182788e+46 2.10571012e+46 1.54494085e+46 1.32654539e+46
1.12621813e+46 8.90637602e+45 7.86853895e+45 5.23696978e+45
4.02194681e+45]
[2.91557514e+46 2.40767289e+46 1.51238108e+46 1.25219489e+46
1.11817366e+46 1.07930148e+46 7.63394887e+45 6.82483337e+45
5.71067068e+45 4.53406700e+45 3.70139527e+45 2.64095805e+45
2.19352087e+45]
[1.72902854e+46 1.38760794e+46 8.55499881e+45 6.57041040e+45
5.52397404e+45 5.02279762e+45 3.36937073e+45 3.05172715e+45
2.73577317e+45 2.34714571e+45 2.21640929e+45 1.54465260e+45
1.26597481e+45]
[9.09250281e+45 7.60481855e+45 4.64708623e+45 3.60128830e+45
3.01048421e+45 2.57240376e+45 1.76179118e+45 1.61750377e+45
1.51045062e+45 1.27696028e+45 1.14758170e+45 9.37847666e+44
7.94380101e+44]
[3.88765612e+45 3.40704126e+45 2.44701133e+45 1.97435341e+45
1.66616691e+45 1.38507901e+45 9.57332778e+44 8.82851837e+44
8.34235667e+44 7.29139573e+44 6.59202667e+44 5.19452977e+44
4.89412860e+44]
[1.60340175e+45 1.19054572e+45 8.15729941e+44 8.35116472e+44
8.33542497e+44 7.67917161e+44 5.63754911e+44 4.80000998e+44
4.50022449e+44 4.22927756e+44 3.55994164e+44 3.21231409e+44
2.92845933e+44]
[5.92187799e+44 4.44857867e+44 4.42250543e+44 4.38381813e+44
4.08428228e+44 4.08529100e+44 2.85836563e+44 2.78447995e+44
2.64411833e+44 2.33564357e+44 2.23469438e+44 2.31885978e+44
1.91987302e+44]
[1.51158672e+44 1.22163223e+44 1.95180187e+44 1.92759872e+44
1.82877352e+44 1.74024175e+44 1.99376976e+44 1.69031210e+44
1.67613850e+44 1.58901094e+44 1.31262249e+44 1.24910409e+44
1.46953387e+44]
[7.38778121e+43 7.93761149e+43 1.48569271e+44 1.43589006e+44
1.30672388e+44 1.22645287e+44 1.21110129e+44 1.18857992e+44
1.08754328e+44 9.82847338e+43 1.03239359e+44 9.70218402e+43
9.23003510e+43]
[3.43885762e+43 5.56151149e+43 9.90184092e+43 9.91721735e+43
9.05236660e+43 8.38760745e+43 7.91949539e+43 7.93646292e+43
7.94932135e+43 8.26036456e+43 7.19649271e+43 6.81132122e+43
5.95602639e+43]
[2.14796226e+43 4.18067995e+43 7.63880271e+43 7.90421952e+43
6.48408551e+43 6.61719399e+43 6.80707574e+43 6.14975888e+43
6.05646668e+43 4.87054339e+43 5.25704353e+43 4.63883781e+43
4.66611594e+43]
[1.28800841e+43 2.71058347e+43 5.47092873e+43 4.95492007e+43
5.72871844e+43 4.33790599e+43 4.19213739e+43 4.23925383e+43
3.57049058e+43 4.41788291e+43 4.78341839e+43 3.43856947e+43
3.65428370e+43]
[7.91278054e+42 1.87879517e+43 3.49278140e+43 3.62872704e+43
3.49918449e+43 2.72408951e+43 2.76562759e+43 3.17330178e+43
2.28362396e+43 2.66438601e+43 2.91662610e+43 2.81341602e+43
2.55406268e+43]
[4.87580023e+42 1.30219192e+43 2.38685588e+43 2.47027813e+43
3.02167043e+43 2.81300642e+43 2.50320240e+43 1.90236559e+43
1.81305456e+43 2.11585791e+43 1.89273210e+43 1.73179565e+43
1.59177903e+43]
[3.34998821e+42 8.66687873e+42 1.55547555e+43 1.53796499e+43
2.09428329e+43 1.62359739e+43 1.33506805e+43 1.92706431e+43
1.33874737e+43 1.44665622e+43 1.25404585e+43 1.18340941e+43
1.18793260e+43]
[1.49236142e+42 4.37738317e+42 7.22336164e+42 8.21596173e+42
9.79382915e+42 9.58059704e+42 1.00484403e+43 1.04013460e+43
7.94320148e+42 8.70000568e+42 9.85813598e+42 7.10497078e+42
7.83250641e+42]
[1.20992856e+42 1.92245219e+42 4.45630473e+42 5.07349773e+42
6.55997954e+42 7.29987268e+42 6.43899189e+42 6.34197193e+42
4.61678419e+42 4.46250670e+42 4.69523651e+42 3.06472534e+42
3.55803773e+42]
[9.69316071e+41 1.14013298e+42 2.15329385e+42 3.70414444e+42
3.58761300e+42 4.67639659e+42 4.34762186e+42 4.15767579e+42
2.79408068e+42 3.25975845e+42 4.33017434e+42 2.27966537e+42
2.67448355e+42]
[4.69704649e+41 5.01743313e+41 1.46178843e+42 1.92821152e+42
2.47026909e+42 2.66896532e+42 2.88264802e+42 2.67634326e+42
1.59280462e+42 1.77617327e+42 1.85619769e+42 1.35118482e+42
1.32850230e+42]
[2.73181573e+41 4.56900368e+41 8.44211376e+41 1.13114903e+42
1.68781021e+42 2.15223146e+42 1.92814838e+42 1.56064696e+42
8.96631090e+41 5.73076573e+41 5.99952619e+41 4.48086391e+41
5.44594749e+41]
[1.57098659e+41 1.92135148e+41 4.52241402e+41 5.03899429e+41
4.64152258e+41 1.00351739e+42 7.67664877e+41 6.99592700e+41
4.26888258e+41 4.07523292e+41 4.93202524e+41 4.06476914e+41
4.51226119e+41]
[9.10691852e+40 1.23416463e+41 2.13210466e+41 2.54386678e+41
2.84039301e+41 5.80973146e+41 5.97338216e+41 4.68960366e+41
2.64212761e+41 2.08436904e+41 2.71978772e+41 2.01419798e+41
2.02507660e+41]
[6.37505468e+40 7.63583695e+40 1.52184338e+41 1.84165552e+41
1.98771322e+41 2.89186554e+41 2.59663518e+41 2.38901461e+41
1.69736989e+41 2.09419668e+41 1.94651955e+41 1.42710349e+41
1.69925377e+41]
[4.49980416e+40 1.03729147e+41 1.10519465e+41 8.90960794e+40
1.08237546e+41 1.37563928e+41 1.45485984e+41 9.64841159e+40
1.20627023e+41 1.07567935e+41 1.41704198e+41 1.60261614e+41
1.77565292e+41]
[5.33866233e+40 5.00464280e+40 1.24895630e+41 9.14079686e+40
7.83281110e+40 8.75956858e+40 9.80087014e+40 6.47583599e+40
3.43316827e+40 9.28119702e+40 7.90440387e+40 1.09274004e+41
1.06687961e+41]
[4.09962265e+40 3.70072898e+40 5.65641508e+40 8.50647486e+40
7.88088943e+40 8.19873804e+40 9.68978237e+40 4.20562302e+40
5.58190043e+40 4.23386846e+40 4.52812155e+40 3.12143883e+40
6.03886136e+40]
[2.51999427e+40 2.57475049e+40 4.65801476e+40 4.97512834e+40
4.78175275e+40 5.79102257e+40 5.73333048e+40 5.64419459e+40
5.77510103e+40 5.57471384e+40 6.71742230e+40 6.81572051e+40
5.51553373e+40]
[2.13704532e+40 2.22405687e+40 3.43065715e+40 3.38590660e+40
3.49770041e+40 3.88015008e+40 4.25197557e+40 3.85699448e+40
4.52559590e+40 6.09634500e+40 5.25792615e+40 6.24928598e+40
6.77556379e+40]
[1.85610004e+40 1.66772993e+40 2.04457747e+40 2.69275980e+40
2.62116237e+40 2.85694389e+40 3.11066233e+40 2.99872401e+40
3.05734665e+40 3.80109423e+40 4.29125745e+40 5.29041168e+40
4.31105878e+40]
[2.13841256e+40 1.59422035e+40 1.86642217e+40 1.55329973e+40
1.88367352e+40 2.00823017e+40 2.09981237e+40 2.75864206e+40
2.77939176e+40 2.93503379e+40 3.24350685e+40 3.78203965e+40
4.91973205e+40]
[7.35261744e+39 6.37610596e+39 9.65035022e+39 1.54707305e+40
1.64962648e+40 1.72443044e+40 1.59263535e+40 1.90833074e+40
2.45993216e+40 2.10601389e+40 2.96142469e+40 3.17923284e+40
3.35676254e+40]
[1.83623291e+40 9.74583222e+39 9.24473595e+39 1.06039514e+40
9.89568395e+39 9.34125084e+39 1.54578793e+40 1.64717193e+40
1.33806584e+40 1.79311294e+40 1.99546931e+40 2.13551881e+40
2.42928069e+40]
[1.42197129e+40 1.10231794e+40 9.45177347e+39 6.56948633e+39
7.78391802e+39 1.12264094e+40 1.01284136e+40 9.92602763e+39
1.34280651e+40 1.56833520e+40 1.30482169e+40 1.92617387e+40
8.23463423e+38]
[3.12953904e+39 4.86120964e+39 5.29646020e+39 8.82982492e+39
8.77236541e+39 8.90362454e+39 7.48056976e+39 9.01382885e+39
1.06577093e+40 9.06533847e+39 1.38888192e+40 1.27467973e+40
4.02747507e+41]
[2.09227154e+40 1.10565491e+40 7.19469242e+39 5.27113321e+39
6.19269500e+39 4.40417535e+39 8.70598116e+39 8.70817762e+39
7.75791895e+39 9.30046413e+39 9.04934353e+39 1.10984911e+39
3.52321683e+41]
[2.91355997e+39 2.56546752e+39 2.79066767e+39 5.12278917e+39
6.75161727e+39 7.31699005e+39 6.39088014e+39 6.69458621e+39
8.06322572e+39 7.80926019e+39 7.66224366e+39 2.10591281e+38
2.02925045e+41]
[3.41533045e+38 1.04154595e+39 3.33345311e+39 4.38093278e+39
3.48616766e+39 3.70803270e+39 4.66644768e+39 5.33471872e+39
5.80965971e+39 6.64710189e+39 2.97730972e+39 1.46585372e+41
2.14168609e+41]
[8.70166606e+38 1.36881998e+39 2.15001445e+39 2.47392479e+39
2.67556818e+39 4.32402714e+39 5.24480860e+39 5.38602933e+39
5.93086204e+39 5.57045556e+39 1.29710520e+37 2.57627418e+41
1.40092058e+41]
[1.76607857e+39 3.75251305e+39 2.05128764e+39 2.17169215e+39
2.38451228e+39 2.62503163e+39 2.91206130e+39 3.09483376e+39
3.20027237e+39 1.97344094e+39 7.54475461e+40 3.03030624e+41
6.59050275e+40]
[1.26504055e+40 8.21469980e+39 1.09865435e+40 3.63469918e+39
2.08049176e+39 2.30439575e+39 2.58669375e+39 2.75696835e+39
1.38021800e+39 4.82344416e+35 2.15432825e+41 1.20314849e+41
5.45087085e+40]
[1.89079354e+41 1.01849506e+41 4.55001746e+40 3.25991557e+40
2.20706938e+39 1.94095890e+39 1.97839461e+39 2.62380467e+36
1.06997603e+35 7.49895924e+40 2.02318050e+41 1.21693461e+41
1.34620674e+41]
[2.85117097e+41 2.44606659e+41 2.08805260e+41 8.92681964e+40
4.32415634e+40 2.42694920e+37 2.54521498e+38 1.84612682e+40
6.17353623e+40 2.02336596e+41 1.45677857e+41 4.86520813e+40
3.60801978e+40]
[7.00896667e+39 1.06831321e+41 2.25801515e+41 1.70855275e+41
1.81355787e+41 6.74537061e+40 5.06977601e+40 2.38078082e+41
1.04061203e+41 1.63107165e+41 7.19859485e+40 8.45408340e+40
8.31498848e+39]
[1.33288015e+37 8.32022846e+40 1.85084557e+30 7.19927698e+40
4.00703180e+37 1.00786613e+41 9.42218927e+40 9.37812865e+40
7.29795495e+40 6.62439504e+40 5.33437671e+40 6.43573527e+40
5.56070570e+39]] 1/s
Combining SEDs¶
Sed
objects can be combined either via concatenation, to produce a single Sed
holding multiple spectra, or by addition, to add the spectra contained in the input Sed
objects.
To concatenate spectra we use Sed.concat()
. Sed.concat
can take an arbitrary number of Sed
objects to combine.
[26]:
print("Shapes before:", sed._lnu.shape, sed2._lnu.shape)
sed3 = sed2.concat(sed)
print("Combined shape:", sed3._lnu.shape)
sed4 = sed2.concat(sed, sed2, sed3)
print("Combined shape:", sed4._lnu.shape)
Shapes before: (9244,) (2, 9244)
Combined shape: (3, 9244)
Combined shape: (8, 9244)
If we want to add the spectra of two Sed
objects together, we simply apply the +
operator. However, unlike concat
, this will only work for Sed
s with identical shapes.
[27]:
sed_add = sed + sed
plt.plot(np.log10(sed.lam), np.log10(sed.lnu), label="sed")
plt.plot(np.log10(sed_add.lam), np.log10(sed_add.lnu), label="sed5")
plt.ylim(26, 30)
plt.xlim(2, 5)
plt.xlabel(r"$\rm log_{10}(\lambda/\AA)$")
plt.ylabel(r"$\rm log_{10}(L_{\nu}/erg\ s^{-1}\ Hz^{-1} M_{\odot}^{-1})$")
plt.legend()
plt.show()
plt.close()
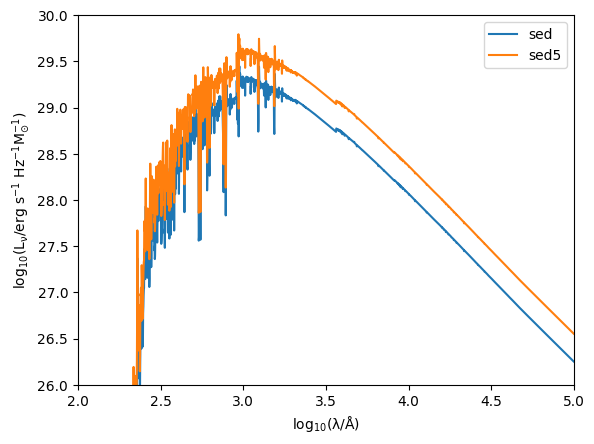
Resampling SEDs¶
The Sed
includes a method to resample an sed, e.g. to lower-resolution or to match some target resolution (e.g. from observations).
[28]:
sed_resampled = sed.get_resampled_sed(5)
plt.plot(np.log10(sed.lam), np.log10(sed.lnu), label="Original")
plt.plot(
np.log10(sed_resampled.lam), np.log10(sed_resampled.lnu), label="Resampled"
)
plt.xlim(2.2, 3.5)
plt.ylim(27.0, 29.5)
plt.xlabel(r"$\rm log_{10}(\lambda/\AA)$")
plt.ylabel(r"$\rm log_{10}(L_{\nu}/erg\ s^{-1}\ Hz^{-1} M_{\odot}^{-1})$")
plt.legend()
plt.show()
plt.close()
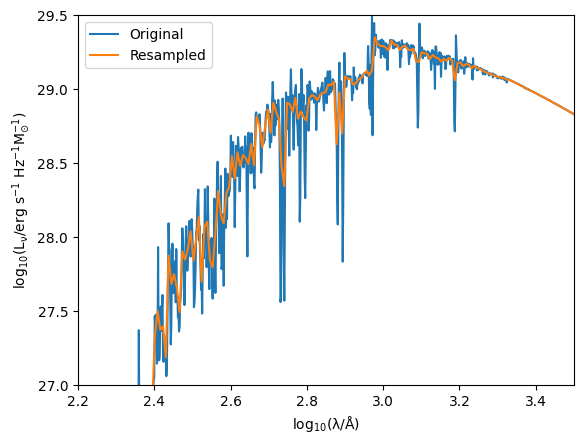
[29]:
print(
sed.measure_bolometric_luminosity() / sed3.measure_bolometric_luminosity()
)
[1. 0.5 1. ] dimensionless
Applying attenuation¶
To apply attenuation to an Sed
you can use the apply_attenuation
method and pass the optical depth and a dust curve (see Attenuation for more details on dust curves).
[30]:
from synthesizer.emission_models.attenuation import PowerLaw
sed4_att = sed4.apply_attenuation(tau_v=0.7, dust_curve=PowerLaw(-1.0))
plt.plot(np.log10(sed4.lam), np.log10(sed4.lnu[0, :]), label="Incident")
plt.plot(
np.log10(sed4_att.lam), np.log10(sed4_att.lnu[0, :]), label="Attenuated"
)
plt.xlim(2.2, 3.5)
plt.ylim(26.0, 30.0)
plt.xlabel(r"$\rm log_{10}(\lambda/\AA)$")
plt.ylabel(r"$\rm log_{10}(L_{\nu}/erg\ s^{-1}\ Hz^{-1} M_{\odot}^{-1})$")
plt.legend()
plt.show()
plt.close()
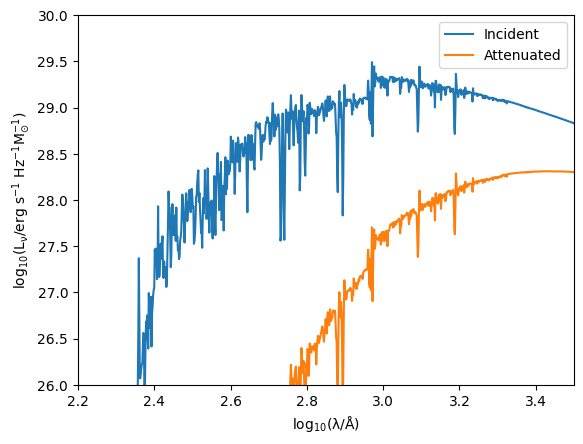
apply_attenuation
can also accept a mask
, which applies attenuation to specific spectra in a multidimensional Sed
(like an Sed
containing the spectra for multiple particles, for instance.)
[31]:
sed0_att = sed4.apply_attenuation(
tau_v=0.7,
dust_curve=PowerLaw(-1.0),
mask=np.array([0, 1, 0, 0, 0, 0, 0, 0], dtype=bool),
)
plt.plot(np.log10(sed4.lam), np.log10(sed4.lnu[1, :]), label="Incident")
plt.plot(np.log10(sed4.lam), np.log10(sed0_att.lnu[0, :]), label="Attenuated")
plt.xlim(2.2, 3.5)
plt.ylim(26.0, 30.0)
plt.xlabel(r"$\rm log_{10}(\lambda/\AA)$")
plt.ylabel(r"$\rm log_{10}(L_{\nu}/erg\ s^{-1}\ Hz^{-1} M_{\odot}^{-1})$")
plt.legend()
plt.show()
plt.close()
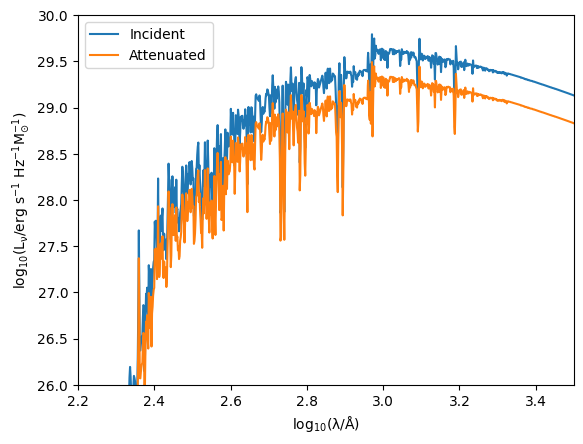
Calculating transmission¶
If you have an attenuated SED, a natural quantity to calculate is the extinction of that spectra (\(A\)). This can be done either at the wavelengths of the Sed
, an arbitrary wavelength/wavelength array, or at commonly used values (1500 and 5500 angstrom) using functions available in the sed
module. Note that these functions return the extinction/attenuation in magnitudes. Below is a demonstration.
[32]:
from unyt import angstrom, um
from synthesizer.sed import (
get_attenuation,
get_attenuation_at_1500,
get_attenuation_at_5500,
get_attenuation_at_lam,
)
# Get an intrinsic spectra
grid_point = grid.get_grid_point(log10ages=7, metallicity=0.01)
int_sed = grid.get_spectra(grid_point, spectra_id="incident")
# Apply an attenuation curve
att_sed = int_sed.apply_attenuation(tau_v=0.7, dust_curve=PowerLaw(-1.0))
# Get attenuation at sed.lam
attenuation = get_attenuation(int_sed, att_sed)
# Get attenuation at 5 microns
att_at_5 = get_attenuation_at_lam(5 * um, int_sed, att_sed)
# Get attenuation at an arbitrary range of wavelengths
att_at_range = get_attenuation_at_lam(
np.linspace(5000, 10000, 5) * angstrom, int_sed, att_sed
)
# Get attenuation at 1500 angstrom
att_at_1500 = get_attenuation_at_1500(int_sed, att_sed)
# Get attenuation at 5500 angstrom
att_at_5500 = get_attenuation_at_5500(int_sed, att_sed)