Abundances¶
Synthesizer can be used to generate abundance patterns, in total and for gas and dust individually, for a given metallicity, alpha ehancement, and arbitrary element scalings.
At present this functionality is only utilised when creating cloudy input scripts. These scripts are used to calculate nebular line and continuum emission for a given incident spectral energy distribution and gas abundance pattern.
[1]:
import matplotlib.pyplot as plt
import numpy as np
from synthesizer.abundances import (
Abundances,
abundance_scalings,
depletion_models,
plot_abundance_pattern,
plot_multiple_abundance_patterns,
reference_abundance_patterns,
)
by default initialising Abundances
creates a solar abundance pattern with no depletion. The default solar abundance pattern is Asplund et al. (2009), though this can be changed if desired.
[2]:
a0 = Abundances()
isinstance(Abundances, type)
[2]:
True
like most synthesizer objects we can explore the important attributes of an object by using print()
:
[3]:
print(a0)
--------------------
ABUNDANCE PATTERN SUMMARY
X: 0.710
Y: 0.275
Z: 0.015
Z/Z_ref: 1
alpha: 0.000
dust mass fraction: 0.0
dust-to-metal ratio: 0.0
----------
Element log10(X/H) log10(X/H)+12 [X/H] depletion log10(X/H)_gas log10(X/H)_dust
hydrogen 0.00 12.00 0.00 1.00 0.00 -inf
helium -1.01 10.99 0.08 1.00 -1.01 -inf
lithium -8.70 3.30 0.02 1.00 -8.70 -inf
beryllium -10.66 1.34 0.02 1.00 -10.66 -inf
boron -9.17 2.83 0.02 1.00 -9.17 -inf
carbon -3.56 8.44 0.02 1.00 -3.56 -inf
nitrogen -4.19 7.81 0.02 1.00 -4.19 -inf
oxygen -3.22 8.78 0.02 1.00 -3.22 -inf
fluorine -7.54 4.46 0.02 1.00 -7.54 -inf
neon -3.89 8.11 0.02 1.00 -3.89 -inf
sodium -5.77 6.23 0.02 1.00 -5.77 -inf
magnesium -4.42 7.58 0.02 1.00 -4.42 -inf
aluminium -5.55 6.45 0.02 1.00 -5.55 -inf
silicon -4.48 7.52 0.02 1.00 -4.48 -inf
phosphorus -6.57 5.43 0.02 1.00 -6.57 -inf
sulphur -4.86 7.14 0.02 1.00 -4.86 -inf
chlorine -6.73 5.27 0.02 1.00 -6.73 -inf
argon -5.58 6.42 0.02 1.00 -5.58 -inf
potassium -6.94 5.06 0.02 1.00 -6.94 -inf
calcium -5.66 6.34 0.02 1.00 -5.66 -inf
scandium -8.82 3.18 0.02 1.00 -8.82 -inf
titanium -7.05 4.95 0.02 1.00 -7.05 -inf
vanadium -8.09 3.91 0.02 1.00 -8.09 -inf
chromium -6.36 5.64 0.02 1.00 -6.36 -inf
manganese -6.56 5.44 0.02 1.00 -6.56 -inf
iron -4.46 7.54 0.02 1.00 -4.46 -inf
cobalt -7.05 4.95 0.02 1.00 -7.05 -inf
nickel -5.78 6.22 0.02 1.00 -5.78 -inf
copper -7.80 4.20 0.02 1.00 -7.80 -inf
zinc -7.42 4.58 0.02 1.00 -7.42 -inf
--------------------
You can access the logarithmic abundances (\(\log_{10}(N_X/N_H)\)) of an element like this:
[4]:
print(f"log10(O/H): {a0.total['O']:.2f}")
print(f"log10(O/H): {a0['O']:.2f}")
log10(O/H): -3.22
log10(O/H): -3.22
Solar abundance pattern¶
As noted, there are several reference abundance patterns built into synthesizer. These can be accessed from synthesizer.abundances.reference_abundance_patterns
:
[5]:
reference_abundance_patterns.available_patterns
[5]:
['Asplund2009', 'GalacticConcordance', 'Gutkin2016']
[6]:
reference = reference_abundance_patterns.Asplund2009()
reference.ads
reference.abundance
[6]:
{'H': 0.0,
'He': -1.07,
'Li': -10.95,
'Be': -10.62,
'B': -9.3,
'C': -3.57,
'N': -4.17,
'O': -3.31,
'F': -7.44,
'Ne': -4.07,
'Na': -5.07,
'Mg': -4.4,
'Al': -5.55,
'Si': -4.49,
'P': -6.59,
'S': -4.88,
'Cl': -6.5,
'Ar': -5.6,
'K': -6.97,
'Ca': -5.66,
'Sc': -8.85,
'Ti': -7.05,
'V': -8.07,
'Cr': -6.36,
'Mn': -6.57,
'Fe': -4.5,
'Co': -7.01,
'Ni': -5.78,
'Cu': -7.81,
'Zn': -7.44}
[7]:
a1 = Abundances(reference=reference_abundance_patterns.Gutkin2016)
print(a1)
--------------------
ABUNDANCE PATTERN SUMMARY
X: 0.709
Y: 0.276
Z: 0.015
Z/Z_ref: 1
alpha: 0.000
dust mass fraction: 0.0
dust-to-metal ratio: 0.0
----------
Element log10(X/H) log10(X/H)+12 [X/H] depletion log10(X/H)_gas log10(X/H)_dust
hydrogen 0.00 12.00 0.00 1.00 0.00 -inf
helium -1.01 10.99 -0.00 1.00 -1.01 -inf
lithium -10.99 1.01 0.00 1.00 -10.99 -inf
beryllium -10.63 1.37 0.00 1.00 -10.63 -inf
boron -9.47 2.53 0.00 1.00 -9.47 -inf
carbon -3.53 8.47 0.00 1.00 -3.53 -inf
nitrogen -4.32 7.68 0.00 1.00 -4.32 -inf
oxygen -3.17 8.83 0.00 1.00 -3.17 -inf
fluorine -7.44 4.56 0.00 1.00 -7.44 -inf
neon -4.01 7.99 0.00 1.00 -4.01 -inf
sodium -5.70 6.30 0.00 1.00 -5.70 -inf
magnesium -4.45 7.55 0.00 1.00 -4.45 -inf
aluminium -5.56 6.44 0.00 1.00 -5.56 -inf
silicon -4.48 7.52 0.00 1.00 -4.48 -inf
phosphorus -6.57 5.43 0.00 1.00 -6.57 -inf
sulphur -4.87 7.13 0.00 1.00 -4.87 -inf
chlorine -6.53 5.47 0.00 1.00 -6.53 -inf
argon -5.63 6.37 0.00 1.00 -5.63 -inf
potassium -6.92 5.08 0.00 1.00 -6.92 -inf
calcium -5.67 6.33 0.00 1.00 -5.67 -inf
scandium -8.86 3.14 0.00 1.00 -8.86 -inf
titanium -7.01 4.99 0.00 1.00 -7.01 -inf
vanadium -8.03 3.97 0.00 1.00 -8.03 -inf
chromium -6.36 5.64 0.00 1.00 -6.36 -inf
manganese -6.64 5.36 0.00 1.00 -6.64 -inf
iron -4.51 7.49 0.00 1.00 -4.51 -inf
cobalt -7.11 4.89 0.00 1.00 -7.11 -inf
nickel -5.78 6.22 0.00 1.00 -5.78 -inf
copper -7.82 4.18 0.00 1.00 -7.82 -inf
zinc -7.43 4.57 0.00 1.00 -7.43 -inf
--------------------
Solar abundance classes can also be called using a string representation of the name.
[8]:
a1 = Abundances(reference="Gutkin2016")
print(a1)
--------------------
ABUNDANCE PATTERN SUMMARY
X: 0.709
Y: 0.276
Z: 0.015
Z/Z_ref: 1
alpha: 0.000
dust mass fraction: 0.0
dust-to-metal ratio: 0.0
----------
Element log10(X/H) log10(X/H)+12 [X/H] depletion log10(X/H)_gas log10(X/H)_dust
hydrogen 0.00 12.00 0.00 1.00 0.00 -inf
helium -1.01 10.99 -0.00 1.00 -1.01 -inf
lithium -10.99 1.01 0.00 1.00 -10.99 -inf
beryllium -10.63 1.37 0.00 1.00 -10.63 -inf
boron -9.47 2.53 0.00 1.00 -9.47 -inf
carbon -3.53 8.47 0.00 1.00 -3.53 -inf
nitrogen -4.32 7.68 0.00 1.00 -4.32 -inf
oxygen -3.17 8.83 0.00 1.00 -3.17 -inf
fluorine -7.44 4.56 0.00 1.00 -7.44 -inf
neon -4.01 7.99 0.00 1.00 -4.01 -inf
sodium -5.70 6.30 0.00 1.00 -5.70 -inf
magnesium -4.45 7.55 0.00 1.00 -4.45 -inf
aluminium -5.56 6.44 0.00 1.00 -5.56 -inf
silicon -4.48 7.52 0.00 1.00 -4.48 -inf
phosphorus -6.57 5.43 0.00 1.00 -6.57 -inf
sulphur -4.87 7.13 0.00 1.00 -4.87 -inf
chlorine -6.53 5.47 0.00 1.00 -6.53 -inf
argon -5.63 6.37 0.00 1.00 -5.63 -inf
potassium -6.92 5.08 0.00 1.00 -6.92 -inf
calcium -5.67 6.33 0.00 1.00 -5.67 -inf
scandium -8.86 3.14 0.00 1.00 -8.86 -inf
titanium -7.01 4.99 0.00 1.00 -7.01 -inf
vanadium -8.03 3.97 0.00 1.00 -8.03 -inf
chromium -6.36 5.64 0.00 1.00 -6.36 -inf
manganese -6.64 5.36 0.00 1.00 -6.64 -inf
iron -4.51 7.49 0.00 1.00 -4.51 -inf
cobalt -7.11 4.89 0.00 1.00 -7.11 -inf
nickel -5.78 6.22 0.00 1.00 -5.78 -inf
copper -7.82 4.18 0.00 1.00 -7.82 -inf
zinc -7.43 4.57 0.00 1.00 -7.43 -inf
--------------------
Metallicity¶
We can specify a different metallicity. By default abundances are scaled from the Solar abundances provided through an optional argument (default Asplund et a. 2009). However, as we will see later, it is possible to set a different \(\alpha\)-enhancement or set arbitrary element scalings.
[9]:
a2 = Abundances(metallicity=0.01)
print(a2)
--------------------
ABUNDANCE PATTERN SUMMARY
X: 0.724
Y: 0.266
Z: 0.010
Z/Z_ref: 0.67
alpha: 0.000
dust mass fraction: 0.0
dust-to-metal ratio: 0.0
----------
Element log10(X/H) log10(X/H)+12 [X/H] depletion log10(X/H)_gas log10(X/H)_dust
hydrogen 0.00 12.00 0.00 1.00 0.00 -inf
helium -1.04 10.96 0.05 1.00 -1.04 -inf
lithium -8.89 3.11 -0.16 1.00 -8.89 -inf
beryllium -10.84 1.16 -0.16 1.00 -10.84 -inf
boron -9.36 2.64 -0.16 1.00 -9.36 -inf
carbon -3.74 8.26 -0.16 1.00 -3.74 -inf
nitrogen -4.37 7.63 -0.16 1.00 -4.37 -inf
oxygen -3.40 8.60 -0.16 1.00 -3.40 -inf
fluorine -7.72 4.28 -0.16 1.00 -7.72 -inf
neon -4.07 7.93 -0.16 1.00 -4.07 -inf
sodium -5.95 6.05 -0.16 1.00 -5.95 -inf
magnesium -4.60 7.40 -0.16 1.00 -4.60 -inf
aluminium -5.73 6.27 -0.16 1.00 -5.73 -inf
silicon -4.66 7.34 -0.16 1.00 -4.66 -inf
phosphorus -6.75 5.25 -0.16 1.00 -6.75 -inf
sulphur -5.04 6.96 -0.16 1.00 -5.04 -inf
chlorine -6.91 5.09 -0.16 1.00 -6.91 -inf
argon -5.76 6.24 -0.16 1.00 -5.76 -inf
potassium -7.12 4.88 -0.16 1.00 -7.12 -inf
calcium -5.84 6.16 -0.16 1.00 -5.84 -inf
scandium -9.00 3.00 -0.16 1.00 -9.00 -inf
titanium -7.23 4.77 -0.16 1.00 -7.23 -inf
vanadium -8.27 3.73 -0.16 1.00 -8.27 -inf
chromium -6.54 5.46 -0.16 1.00 -6.54 -inf
manganese -6.74 5.26 -0.16 1.00 -6.74 -inf
iron -4.64 7.36 -0.16 1.00 -4.64 -inf
cobalt -7.23 4.77 -0.16 1.00 -7.23 -inf
nickel -5.96 6.04 -0.16 1.00 -5.96 -inf
copper -7.98 4.02 -0.16 1.00 -7.98 -inf
zinc -7.60 4.40 -0.16 1.00 -7.60 -inf
--------------------
\(\alpha\)-enhancement¶
We can also generate abundance patterns assuming different \(\alpha\)-enhancements. In this case it is necessary to re-scale the non-\(\alpha\) elements to recover the input metallicity.
[10]:
a3 = Abundances(alpha=0.6)
print(a3)
--------------------
ABUNDANCE PATTERN SUMMARY
X: 0.710
Y: 0.275
Z: 0.015
Z/Z_ref: 1
alpha: 0.600
dust mass fraction: 0.0
dust-to-metal ratio: 0.0
----------
Element log10(X/H) log10(X/H)+12 [X/H] depletion log10(X/H)_gas log10(X/H)_dust
hydrogen 0.00 12.00 0.00 1.00 0.00 -inf
helium -1.01 10.99 0.08 1.00 -1.01 -inf
lithium -9.19 2.81 -0.47 1.00 -9.19 -inf
beryllium -11.15 0.85 -0.47 1.00 -11.15 -inf
boron -9.66 2.34 -0.47 1.00 -9.66 -inf
carbon -4.04 7.96 -0.47 1.00 -4.04 -inf
nitrogen -4.68 7.32 -0.47 1.00 -4.68 -inf
oxygen -3.11 8.89 0.13 1.00 -3.11 -inf
fluorine -8.03 3.97 -0.47 1.00 -8.03 -inf
neon -3.78 8.22 0.13 1.00 -3.78 -inf
sodium -6.26 5.74 -0.47 1.00 -6.26 -inf
magnesium -4.31 7.69 0.13 1.00 -4.31 -inf
aluminium -6.04 5.96 -0.47 1.00 -6.04 -inf
silicon -4.37 7.63 0.13 1.00 -4.37 -inf
phosphorus -7.06 4.94 -0.47 1.00 -7.06 -inf
sulphur -4.75 7.25 0.13 1.00 -4.75 -inf
chlorine -7.22 4.78 -0.47 1.00 -7.22 -inf
argon -5.47 6.53 0.13 1.00 -5.47 -inf
potassium -7.43 4.57 -0.47 1.00 -7.43 -inf
calcium -5.55 6.45 0.13 1.00 -5.55 -inf
scandium -9.31 2.69 -0.47 1.00 -9.31 -inf
titanium -6.94 5.06 0.13 1.00 -6.94 -inf
vanadium -8.58 3.42 -0.47 1.00 -8.58 -inf
chromium -6.85 5.15 -0.47 1.00 -6.85 -inf
manganese -7.05 4.95 -0.47 1.00 -7.05 -inf
iron -4.95 7.05 -0.47 1.00 -4.95 -inf
cobalt -7.54 4.46 -0.47 1.00 -7.54 -inf
nickel -6.27 5.73 -0.47 1.00 -6.27 -inf
copper -8.29 3.71 -0.47 1.00 -8.29 -inf
zinc -7.91 4.09 -0.47 1.00 -7.91 -inf
--------------------
We can print a relative solar abundance like this:
[11]:
print(f"[O/Fe] = {a3.reference_relative_abundance('O', ref_element='Fe'):.2f}")
print(f"[O/Fe] = {a3['[O/Fe]']:.2f}")
print(f"[B/Fe] = {a3['[B/Fe]']:.2f}")
[O/Fe] = 0.60
[O/Fe] = 0.60
[B/Fe] = 0.00
That, is, as expected given that we set \(\alpha=0.6\).
Depletion¶
To account for metals being locked up in dust, we can also specify a depletion pattern. It is possible to either provide a dictionary of values or specify one of the in-built patterns.
[12]:
# assume 99% of Carbon and Iron are depleted on to dust
depletion = {"C": 0.99, "Fe": 0.99}
# calculate the abundance patterns, now included gas and dust separately
a4 = Abundances(metallicity=0.01, depletion=depletion)
print(a4)
--------------------
ABUNDANCE PATTERN SUMMARY
X: 0.724
Y: 0.266
Z: 0.010
Z/Z_ref: 0.67
alpha: 0.000
dust mass fraction: 2.486382257427241e-05
dust-to-metal ratio: 0.002486382257427241
----------
Element log10(X/H) log10(X/H)+12 [X/H] depletion log10(X/H)_gas log10(X/H)_dust
hydrogen 0.00 12.00 0.00 1.00 0.00 -inf
helium -1.04 10.96 0.05 1.00 -1.04 -inf
lithium -8.89 3.11 -0.16 1.00 -8.89 -inf
beryllium -10.84 1.16 -0.16 1.00 -10.84 -inf
boron -9.36 2.64 -0.16 1.00 -9.36 -inf
carbon -3.74 8.26 -0.16 0.99 -3.74 -5.74
nitrogen -4.37 7.63 -0.16 1.00 -4.37 -inf
oxygen -3.40 8.60 -0.16 1.00 -3.40 -inf
fluorine -7.72 4.28 -0.16 1.00 -7.72 -inf
neon -4.07 7.93 -0.16 1.00 -4.07 -inf
sodium -5.95 6.05 -0.16 1.00 -5.95 -inf
magnesium -4.60 7.40 -0.16 1.00 -4.60 -inf
aluminium -5.73 6.27 -0.16 1.00 -5.73 -inf
silicon -4.66 7.34 -0.16 1.00 -4.66 -inf
phosphorus -6.75 5.25 -0.16 1.00 -6.75 -inf
sulphur -5.04 6.96 -0.16 1.00 -5.04 -inf
chlorine -6.91 5.09 -0.16 1.00 -6.91 -inf
argon -5.76 6.24 -0.16 1.00 -5.76 -inf
potassium -7.12 4.88 -0.16 1.00 -7.12 -inf
calcium -5.84 6.16 -0.16 1.00 -5.84 -inf
scandium -9.00 3.00 -0.16 1.00 -9.00 -inf
titanium -7.23 4.77 -0.16 1.00 -7.23 -inf
vanadium -8.27 3.73 -0.16 1.00 -8.27 -inf
chromium -6.54 5.46 -0.16 1.00 -6.54 -inf
manganese -6.74 5.26 -0.16 1.00 -6.74 -inf
iron -4.64 7.36 -0.16 0.99 -4.65 -6.64
cobalt -7.23 4.77 -0.16 1.00 -7.23 -inf
nickel -5.96 6.04 -0.16 1.00 -5.96 -inf
copper -7.98 4.02 -0.16 1.00 -7.98 -inf
zinc -7.60 4.40 -0.16 1.00 -7.60 -inf
--------------------
[13]:
a5 = Abundances(
metallicity=0.0156,
reference=reference_abundance_patterns.Gutkin2016,
depletion_model=depletion_models.Gutkin2016,
)
print(a5)
--------------------
ABUNDANCE PATTERN SUMMARY
X: 0.708
Y: 0.276
Z: 0.016
Z/Z_ref: 1
alpha: 0.000
dust mass fraction: 0.0062629182900424815
dust-to-metal ratio: 0.40146912115656935
----------
Element log10(X/H) log10(X/H)+12 [X/H] depletion log10(X/H)_gas log10(X/H)_dust
hydrogen 0.00 12.00 0.00 1.00 0.00 -inf
helium -1.01 10.99 -0.00 1.00 -1.01 -inf
lithium -10.98 1.02 0.01 0.16 -11.77 -11.05
beryllium -10.62 1.38 0.01 0.60 -10.84 -11.01
boron -9.46 2.54 0.01 0.13 -10.34 -9.52
carbon -3.52 8.48 0.01 0.50 -3.82 -3.82
nitrogen -4.31 7.69 0.01 1.00 -4.31 -inf
oxygen -3.16 8.84 0.01 0.70 -3.31 -3.68
fluorine -7.43 4.57 0.01 0.30 -7.95 -7.58
neon -4.00 8.00 0.01 1.00 -4.00 -inf
sodium -5.69 6.31 0.01 0.25 -6.29 -5.81
magnesium -4.44 7.56 0.01 0.20 -5.13 -4.53
aluminium -5.55 6.45 0.01 0.02 -7.24 -5.55
silicon -4.47 7.53 0.01 0.10 -5.47 -4.51
phosphorus -6.56 5.44 0.01 0.25 -7.16 -6.68
sulphur -4.86 7.14 0.01 1.00 -4.86 -inf
chlorine -6.52 5.48 0.01 0.50 -6.82 -6.82
argon -5.62 6.38 0.01 1.00 -5.62 -inf
potassium -6.91 5.09 0.01 0.30 -7.43 -7.06
calcium -5.66 6.34 0.01 0.00 -8.18 -5.66
scandium -8.85 3.15 0.01 0.01 -11.15 -8.85
titanium -7.00 5.00 0.01 0.01 -9.09 -7.00
vanadium -8.02 3.98 0.01 0.01 -10.24 -8.02
chromium -6.35 5.65 0.01 0.01 -8.57 -6.35
manganese -6.63 5.37 0.01 0.05 -7.93 -6.65
iron -4.50 7.50 0.01 0.01 -6.50 -4.50
cobalt -7.10 4.90 0.01 0.01 -9.10 -7.10
nickel -5.77 6.23 0.01 0.04 -7.16 -5.78
copper -7.81 4.19 0.01 0.10 -8.81 -7.85
zinc -7.42 4.58 0.01 0.25 -8.02 -7.54
--------------------
[14]:
a6 = Abundances(
metallicity=0.0156,
reference=reference_abundance_patterns.Gutkin2016,
depletion_model=depletion_models.CloudyClassic,
)
print(a6)
--------------------
ABUNDANCE PATTERN SUMMARY
X: 0.708
Y: 0.276
Z: 0.016
Z/Z_ref: 1
alpha: 0.000
dust mass fraction: 0.007361330259064843
dust-to-metal ratio: 0.4718801448118489
----------
Element log10(X/H) log10(X/H)+12 [X/H] depletion log10(X/H)_gas log10(X/H)_dust
hydrogen 0.00 12.00 0.00 1.00 0.00 -inf
helium -1.01 10.99 -0.00 1.00 -1.01 -inf
lithium -10.98 1.02 0.01 0.16 -11.77 -11.05
beryllium -10.62 1.38 0.01 0.60 -10.84 -11.01
boron -9.46 2.54 0.01 0.13 -10.34 -9.52
carbon -3.52 8.48 0.01 0.40 -3.91 -3.74
nitrogen -4.31 7.69 0.01 1.00 -4.31 -inf
oxygen -3.16 8.84 0.01 0.60 -3.38 -3.55
fluorine -7.43 4.57 0.01 0.30 -7.95 -7.58
neon -4.00 8.00 0.01 1.00 -4.00 -inf
sodium -5.69 6.31 0.01 0.20 -6.38 -5.78
magnesium -4.44 7.56 0.01 0.20 -5.13 -4.53
aluminium -5.55 6.45 0.01 0.01 -7.55 -5.55
silicon -4.47 7.53 0.01 0.03 -5.99 -4.48
phosphorus -6.56 5.44 0.01 0.25 -7.16 -6.68
sulphur -4.86 7.14 0.01 1.00 -4.86 -inf
chlorine -6.52 5.48 0.01 0.40 -6.91 -6.74
argon -5.62 6.38 0.01 1.00 -5.62 -inf
potassium -6.91 5.09 0.01 0.30 -7.43 -7.06
calcium -5.66 6.34 0.01 0.00 -9.66 -5.66
scandium -8.85 3.15 0.01 0.01 -11.15 -8.85
titanium -7.00 5.00 0.01 0.01 -9.09 -7.00
vanadium -8.02 3.98 0.01 0.01 -10.24 -8.02
chromium -6.35 5.65 0.01 0.01 -8.57 -6.35
manganese -6.63 5.37 0.01 0.05 -7.93 -6.65
iron -4.50 7.50 0.01 0.01 -6.50 -4.50
cobalt -7.10 4.90 0.01 0.01 -9.10 -7.10
nickel -5.77 6.23 0.01 0.01 -7.77 -5.77
copper -7.81 4.19 0.01 0.10 -8.81 -7.85
zinc -7.42 4.58 0.01 0.25 -8.02 -7.54
--------------------
[15]:
a7 = Abundances(
metallicity=0.0156,
reference=reference_abundance_patterns.Gutkin2016,
depletion_model=depletion_models.Jenkins2009_Gunasekera2021,
)
print(a7)
--------------------
ABUNDANCE PATTERN SUMMARY
X: 0.708
Y: 0.276
Z: 0.016
Z/Z_ref: 1
alpha: 0.000
dust mass fraction: 0.005665821639073615
dust-to-metal ratio: 0.3631936948124112
----------
Element log10(X/H) log10(X/H)+12 [X/H] depletion log10(X/H)_gas log10(X/H)_dust
hydrogen 0.00 12.00 0.00 1.00 0.00 -inf
helium -1.01 10.99 -0.00 1.00 -1.01 -inf
lithium -10.98 1.02 0.01 0.15 -11.79 -11.05
beryllium -10.62 1.38 0.01 1.00 -10.62 -inf
boron -9.46 2.54 0.01 1.00 -9.46 -inf
carbon -3.52 8.48 0.01 0.69 -3.68 -4.02
nitrogen -4.31 7.69 0.01 0.78 -4.42 -4.96
oxygen -3.16 8.84 0.01 0.75 -3.28 -3.75
fluorine -7.43 4.57 0.01 1.00 -7.43 -inf
neon -4.00 8.00 0.01 1.00 -4.00 -inf
sodium -5.69 6.31 0.01 0.01 -7.71 -5.69
magnesium -4.44 7.56 0.01 0.17 -5.21 -4.52
aluminium -5.55 6.45 0.01 0.03 -7.03 -5.56
silicon -4.47 7.53 0.01 0.16 -5.25 -4.54
phosphorus -6.56 5.44 0.01 0.66 -6.73 -7.03
sulphur -4.86 7.14 0.01 0.53 -5.13 -5.18
chlorine -6.52 5.48 0.01 0.67 -6.69 -7.00
argon -5.62 6.38 0.01 0.41 -6.01 -5.84
potassium -6.91 5.09 0.01 0.12 -7.83 -6.96
calcium -5.66 6.34 0.01 0.00 -8.33 -5.66
scandium -8.85 3.15 0.01 1.00 -8.85 -inf
titanium -7.00 5.00 0.01 0.01 -9.10 -7.00
vanadium -8.02 3.98 0.01 1.00 -8.02 -inf
chromium -6.35 5.65 0.01 0.03 -7.90 -6.36
manganese -6.63 5.37 0.01 0.05 -7.96 -6.65
iron -4.50 7.50 0.01 0.03 -6.08 -4.51
cobalt -7.10 4.90 0.01 1.00 -7.10 -inf
nickel -5.77 6.23 0.01 0.02 -7.45 -5.77
copper -7.81 4.19 0.01 0.11 -8.76 -7.86
zinc -7.42 4.58 0.01 0.57 -7.66 -7.78
--------------------
When specifying an in-built pattern it’s also possible to specify an optional scaling parameter depending on the particular model.
Below we explore the effect of \(F_{*}\) on the depletion factors for N, O, and S.
[16]:
for element in ["N", "O", "S"]:
print(element, "-" * 5)
for fstar in [0.0, 0.5, 1.0]:
depletion = depletion_models.Jenkins2009_Gunasekera2021(
fstar
).depletion[element]
print(f"{fstar} {depletion:.2f} {np.log10(depletion):.2f}")
N -----
0.0 0.78 -0.11
0.5 0.78 -0.11
1.0 0.78 -0.11
O -----
0.0 0.97 -0.01
0.5 0.75 -0.13
1.0 0.57 -0.24
S -----
0.0 1.00 0.00
0.5 0.53 -0.27
1.0 0.19 -0.71
Next, we explore the effect of \(F_{*}\) on the dust mass fraction and dust-to-metal ratio (\(\xi_{d}\)):
[17]:
print("F_*", "dust_abundance", "dust_mass_fraction", "dust_to_metal_ratio")
for fstar in [0.0, 0.1, 0.25, 0.5, 1.0]:
a = Abundances(
metallicity=0.0156,
reference=reference_abundance_patterns.Gutkin2016,
depletion_model=depletion_models.Jenkins2009_Gunasekera2021,
depletion_scale=fstar,
)
print(
fstar,
f"{a.dust_abundance:.2g}",
f"{a.dust_mass_fraction:.2g}",
f"{a.dust_to_metal_ratio:.2g}",
)
F_* dust_abundance dust_mass_fraction dust_to_metal_ratio
0.0 0.00017 0.0028 0.18
0.1 0.00022 0.0035 0.22
0.25 0.00029 0.0044 0.28
0.5 0.00039 0.0057 0.36
1.0 0.00055 0.0075 0.48
We can also adjust the depletion scale in place and recalculate the dust mass:
[18]:
a = Abundances(
metallicity=0.0156,
reference=reference_abundance_patterns.Gutkin2016,
depletion_model=depletion_models.Jenkins2009_Gunasekera2021,
depletion_scale=0.5,
)
print(f"{a.dust_abundance:.2g}")
a.add_depletion(
depletion_model="Jenkins2009_Gunasekera2021", depletion_scale=1.0
)
print(f"{a.dust_abundance:.2g}")
a.add_depletion(
depletion_model="Jenkins2009_Gunasekera2021", depletion_scale=0.5
)
print(f"{a.dust_abundance:.2g}")
0.00039
0.00055
0.00039
[19]:
# define default model
a = Abundances(
metallicity=0.0156,
reference=reference_abundance_patterns.Gutkin2016,
depletion_model=depletion_models.Jenkins2009_Gunasekera2021,
depletion_scale=0.5,
)
default_dust_mass_fraction = a.dust_mass_fraction
# vary fstar and recalaculate the dust_mass_fraction
for fstar in [0.0, 0.1, 0.25, 0.5, 1.0]:
# recalcualte for different fstar
a.add_depletion(
depletion_model="Jenkins2009_Gunasekera2021", depletion_scale=fstar
)
dust_mass_fraction = a.dust_mass_fraction
print(
f"""{fstar} {default_dust_mass_fraction:.4f} {dust_mass_fraction:.4f}
{dust_mass_fraction/default_dust_mass_fraction:.2f}"""
)
0.0 0.0057 0.0028
0.49
0.1 0.0057 0.0035
0.61
0.25 0.0057 0.0044
0.77
0.5 0.0057 0.0057
1.00
1.0 0.0057 0.0075
1.33
When the depletion is applied the total, gas, and dust abundance patterns are provided e.g.
[20]:
print(f'log10(C_total/H) : {a7.total["C"]:.2f}')
print(f'log10(C_gas/H) : {a7.gas["C"]:.2f}')
print(f'log10(C_dust/H) : {a7.dust["C"]:.2f}')
log10(C_total/H) : -3.52
log10(C_gas/H) : -3.68
log10(C_dust/H) : -4.02
Arbitrary element scaling¶
We can also change the abundance of any specific element (or set of elements), with the abundances of other elements rescaled self-consistently to yield the correct metallicity.
If the abundance is a float it is the logarithmic abundance (\(\log_{10}(X/H)\)) while if it is a string it is one of the in-built functions that scale the abundance with metallicity (e.g. the model proposed by Dopita et al. 2006). Note, combining this with a non-zero alpha
can lead to a mild inconsistency.
Using a float for the absolute abundance (relative to H):
[21]:
a8 = Abundances(metallicity=0.0134, abundances={"nitrogen": -4.5})
print(a8)
--------------------
ABUNDANCE PATTERN SUMMARY
X: 0.714
Y: 0.272
Z: 0.013
Z/Z_ref: 0.89
alpha: 0.000
dust mass fraction: 0.0
dust-to-metal ratio: 0.0
----------
Element log10(X/H) log10(X/H)+12 [X/H] depletion log10(X/H)_gas log10(X/H)_dust
hydrogen 0.00 12.00 0.00 1.00 0.00 -inf
helium -1.02 10.98 0.07 1.00 -1.02 -inf
lithium -8.74 3.26 -0.02 1.00 -8.74 -inf
beryllium -10.70 1.30 -0.02 1.00 -10.70 -inf
boron -9.21 2.79 -0.02 1.00 -9.21 -inf
carbon -3.60 8.40 -0.02 1.00 -3.60 -inf
nitrogen -4.50 7.50 -0.29 1.00 -4.50 -inf
oxygen -3.26 8.74 -0.02 1.00 -3.26 -inf
fluorine -7.58 4.42 -0.02 1.00 -7.58 -inf
neon -3.93 8.07 -0.02 1.00 -3.93 -inf
sodium -5.81 6.19 -0.02 1.00 -5.81 -inf
magnesium -4.46 7.54 -0.02 1.00 -4.46 -inf
aluminium -5.59 6.41 -0.02 1.00 -5.59 -inf
silicon -4.52 7.48 -0.02 1.00 -4.52 -inf
phosphorus -6.61 5.39 -0.02 1.00 -6.61 -inf
sulphur -4.90 7.10 -0.02 1.00 -4.90 -inf
chlorine -6.77 5.23 -0.02 1.00 -6.77 -inf
argon -5.62 6.38 -0.02 1.00 -5.62 -inf
potassium -6.98 5.02 -0.02 1.00 -6.98 -inf
calcium -5.70 6.30 -0.02 1.00 -5.70 -inf
scandium -8.86 3.14 -0.02 1.00 -8.86 -inf
titanium -7.09 4.91 -0.02 1.00 -7.09 -inf
vanadium -8.13 3.87 -0.02 1.00 -8.13 -inf
chromium -6.40 5.60 -0.02 1.00 -6.40 -inf
manganese -6.60 5.40 -0.02 1.00 -6.60 -inf
iron -4.50 7.50 -0.02 1.00 -4.50 -inf
cobalt -7.09 4.91 -0.02 1.00 -7.09 -inf
nickel -5.82 6.18 -0.02 1.00 -5.82 -inf
copper -7.84 4.16 -0.02 1.00 -7.84 -inf
zinc -7.46 4.54 -0.02 1.00 -7.46 -inf
--------------------
Providing an abundance relative to another element:
[22]:
a9a = Abundances(metallicity=0.0134, abundances={"nitrogen_to_oxygen": -0.4})
print(a9a)
# same as above but less PEP8 compliant
a9b = Abundances(metallicity=0.0134, abundances={"N/O": -0.4})
print(a9b)
--------------------
ABUNDANCE PATTERN SUMMARY
X: 0.714
Y: 0.272
Z: 0.013
Z/Z_ref: 0.89
alpha: 0.000
dust mass fraction: 0.0
dust-to-metal ratio: 0.0
----------
Element log10(X/H) log10(X/H)+12 [X/H] depletion log10(X/H)_gas log10(X/H)_dust
hydrogen 0.00 12.00 0.00 1.00 0.00 -inf
helium -1.02 10.98 0.07 1.00 -1.02 -inf
lithium -8.80 3.20 -0.08 1.00 -8.80 -inf
beryllium -10.76 1.24 -0.08 1.00 -10.76 -inf
boron -9.27 2.73 -0.08 1.00 -9.27 -inf
carbon -3.65 8.35 -0.08 1.00 -3.65 -inf
nitrogen -3.72 8.28 0.49 1.00 -3.72 -inf
oxygen -3.32 8.68 -0.08 1.00 -3.32 -inf
fluorine -7.64 4.36 -0.08 1.00 -7.64 -inf
neon -3.99 8.01 -0.08 1.00 -3.99 -inf
sodium -5.87 6.13 -0.08 1.00 -5.87 -inf
magnesium -4.52 7.48 -0.08 1.00 -4.52 -inf
aluminium -5.65 6.35 -0.08 1.00 -5.65 -inf
silicon -4.58 7.42 -0.08 1.00 -4.58 -inf
phosphorus -6.67 5.33 -0.08 1.00 -6.67 -inf
sulphur -4.96 7.04 -0.08 1.00 -4.96 -inf
chlorine -6.83 5.17 -0.08 1.00 -6.83 -inf
argon -5.68 6.32 -0.08 1.00 -5.68 -inf
potassium -7.04 4.96 -0.08 1.00 -7.04 -inf
calcium -5.76 6.24 -0.08 1.00 -5.76 -inf
scandium -8.92 3.08 -0.08 1.00 -8.92 -inf
titanium -7.15 4.85 -0.08 1.00 -7.15 -inf
vanadium -8.19 3.81 -0.08 1.00 -8.19 -inf
chromium -6.46 5.54 -0.08 1.00 -6.46 -inf
manganese -6.66 5.34 -0.08 1.00 -6.66 -inf
iron -4.56 7.44 -0.08 1.00 -4.56 -inf
cobalt -7.15 4.85 -0.08 1.00 -7.15 -inf
nickel -5.88 6.12 -0.08 1.00 -5.88 -inf
copper -7.90 4.10 -0.08 1.00 -7.90 -inf
zinc -7.52 4.48 -0.08 1.00 -7.52 -inf
--------------------
--------------------
ABUNDANCE PATTERN SUMMARY
X: 0.714
Y: 0.272
Z: 0.013
Z/Z_ref: 0.89
alpha: 0.000
dust mass fraction: 0.0
dust-to-metal ratio: 0.0
----------
Element log10(X/H) log10(X/H)+12 [X/H] depletion log10(X/H)_gas log10(X/H)_dust
hydrogen 0.00 12.00 0.00 1.00 0.00 -inf
helium -1.02 10.98 0.07 1.00 -1.02 -inf
lithium -8.80 3.20 -0.08 1.00 -8.80 -inf
beryllium -10.76 1.24 -0.08 1.00 -10.76 -inf
boron -9.27 2.73 -0.08 1.00 -9.27 -inf
carbon -3.65 8.35 -0.08 1.00 -3.65 -inf
nitrogen -3.72 8.28 0.49 1.00 -3.72 -inf
oxygen -3.32 8.68 -0.08 1.00 -3.32 -inf
fluorine -7.64 4.36 -0.08 1.00 -7.64 -inf
neon -3.99 8.01 -0.08 1.00 -3.99 -inf
sodium -5.87 6.13 -0.08 1.00 -5.87 -inf
magnesium -4.52 7.48 -0.08 1.00 -4.52 -inf
aluminium -5.65 6.35 -0.08 1.00 -5.65 -inf
silicon -4.58 7.42 -0.08 1.00 -4.58 -inf
phosphorus -6.67 5.33 -0.08 1.00 -6.67 -inf
sulphur -4.96 7.04 -0.08 1.00 -4.96 -inf
chlorine -6.83 5.17 -0.08 1.00 -6.83 -inf
argon -5.68 6.32 -0.08 1.00 -5.68 -inf
potassium -7.04 4.96 -0.08 1.00 -7.04 -inf
calcium -5.76 6.24 -0.08 1.00 -5.76 -inf
scandium -8.92 3.08 -0.08 1.00 -8.92 -inf
titanium -7.15 4.85 -0.08 1.00 -7.15 -inf
vanadium -8.19 3.81 -0.08 1.00 -8.19 -inf
chromium -6.46 5.54 -0.08 1.00 -6.46 -inf
manganese -6.66 5.34 -0.08 1.00 -6.66 -inf
iron -4.56 7.44 -0.08 1.00 -4.56 -inf
cobalt -7.15 4.85 -0.08 1.00 -7.15 -inf
nickel -5.88 6.12 -0.08 1.00 -5.88 -inf
copper -7.90 4.10 -0.08 1.00 -7.90 -inf
zinc -7.52 4.48 -0.08 1.00 -7.52 -inf
--------------------
Alternatively, we can use a built-in function for particular set of elements or for all elements available. For example, here we use the Dopita (2006) scaling relation to adjust Nitrogen.
[23]:
a10 = Abundances(metallicity=0.0134, abundances={"nitrogen": "Dopita2006"})
print(a10)
--------------------
ABUNDANCE PATTERN SUMMARY
X: 0.714
Y: 0.272
Z: 0.013
Z/Z_ref: 0.89
alpha: 0.000
dust mass fraction: 0.0
dust-to-metal ratio: 0.0
----------
Element log10(X/H) log10(X/H)+12 [X/H] depletion log10(X/H)_gas log10(X/H)_dust
hydrogen 0.00 12.00 0.00 1.00 0.00 -inf
helium -1.02 10.98 0.07 1.00 -1.02 -inf
lithium -8.75 3.25 -0.03 1.00 -8.75 -inf
beryllium -10.71 1.29 -0.03 1.00 -10.71 -inf
boron -9.22 2.78 -0.03 1.00 -9.22 -inf
carbon -3.60 8.40 -0.03 1.00 -3.60 -inf
nitrogen -4.36 7.64 -0.15 1.00 -4.36 -inf
oxygen -3.27 8.73 -0.03 1.00 -3.27 -inf
fluorine -7.59 4.41 -0.03 1.00 -7.59 -inf
neon -3.94 8.06 -0.03 1.00 -3.94 -inf
sodium -5.82 6.18 -0.03 1.00 -5.82 -inf
magnesium -4.47 7.53 -0.03 1.00 -4.47 -inf
aluminium -5.60 6.40 -0.03 1.00 -5.60 -inf
silicon -4.53 7.47 -0.03 1.00 -4.53 -inf
phosphorus -6.62 5.38 -0.03 1.00 -6.62 -inf
sulphur -4.91 7.09 -0.03 1.00 -4.91 -inf
chlorine -6.78 5.22 -0.03 1.00 -6.78 -inf
argon -5.63 6.37 -0.03 1.00 -5.63 -inf
potassium -6.99 5.01 -0.03 1.00 -6.99 -inf
calcium -5.71 6.29 -0.03 1.00 -5.71 -inf
scandium -8.87 3.13 -0.03 1.00 -8.87 -inf
titanium -7.10 4.90 -0.03 1.00 -7.10 -inf
vanadium -8.14 3.86 -0.03 1.00 -8.14 -inf
chromium -6.41 5.59 -0.03 1.00 -6.41 -inf
manganese -6.61 5.39 -0.03 1.00 -6.61 -inf
iron -4.51 7.49 -0.03 1.00 -4.51 -inf
cobalt -7.10 4.90 -0.03 1.00 -7.10 -inf
nickel -5.83 6.17 -0.03 1.00 -5.83 -inf
copper -7.85 4.15 -0.03 1.00 -7.85 -inf
zinc -7.47 4.53 -0.03 1.00 -7.47 -inf
--------------------
We can also use a model to set all scalings for all available elements.
[24]:
a11 = Abundances(metallicity=0.015, abundances="GalacticConcordance")
print(a11)
--------------------
ABUNDANCE PATTERN SUMMARY
X: 0.710
Y: 0.275
Z: 0.015
Z/Z_ref: 1
alpha: 0.000
dust mass fraction: 0.0
dust-to-metal ratio: 0.0
----------
Element log10(X/H) log10(X/H)+12 [X/H] depletion log10(X/H)_gas log10(X/H)_dust
hydrogen 0.00 12.00 0.00 1.00 0.00 -inf
helium -1.01 10.99 0.08 1.00 -1.01 -inf
lithium -8.68 3.32 0.05 1.00 -8.68 -inf
beryllium -10.63 1.37 0.05 1.00 -10.63 -inf
boron -9.15 2.85 0.05 1.00 -9.15 -inf
carbon -3.58 8.42 0.00 1.00 -3.58 -inf
nitrogen -4.21 7.79 0.00 1.00 -4.21 -inf
oxygen -3.19 8.81 0.05 1.00 -3.19 -inf
fluorine -7.56 4.44 0.00 1.00 -7.56 -inf
neon -3.91 8.09 0.00 1.00 -3.91 -inf
sodium -5.79 6.21 0.00 1.00 -5.79 -inf
magnesium -4.44 7.56 0.00 1.00 -4.44 -inf
aluminium -5.57 6.43 0.00 1.00 -5.57 -inf
silicon -4.50 7.50 0.00 1.00 -4.50 -inf
phosphorus -6.59 5.41 0.00 1.00 -6.59 -inf
sulphur -4.88 7.12 0.00 1.00 -4.88 -inf
chlorine -6.75 5.25 0.00 1.00 -6.75 -inf
argon -5.60 6.40 0.00 1.00 -5.60 -inf
potassium -6.96 5.04 0.00 1.00 -6.96 -inf
calcium -5.68 6.32 0.00 1.00 -5.68 -inf
scandium -8.84 3.16 0.00 1.00 -8.84 -inf
titanium -7.07 4.93 0.00 1.00 -7.07 -inf
vanadium -8.11 3.89 0.00 1.00 -8.11 -inf
chromium -6.38 5.62 0.00 1.00 -6.38 -inf
manganese -6.58 5.42 0.00 1.00 -6.58 -inf
iron -4.48 7.52 0.00 1.00 -4.48 -inf
cobalt -7.07 4.93 0.00 1.00 -7.07 -inf
nickel -5.80 6.20 0.00 1.00 -5.80 -inf
copper -7.82 4.18 0.00 1.00 -7.82 -inf
zinc -7.44 4.56 0.00 1.00 -7.44 -inf
--------------------
Scaling models¶
Let’s look in a bit more detail at some of the available scaling relationships.
We can also access the scaling functions directly:
[25]:
abundance_scalings.available_scalings
[25]:
['Dopita2006', 'GalacticConcordance']
[26]:
abundance_scalings.GalacticConcordance().available_elements
[26]:
['N',
'C',
'F',
'Ne',
'Na',
'Mg',
'Al',
'Si',
'P',
'S',
'Cl',
'Ar',
'K',
'Ca',
'Sc',
'Ti',
'V',
'Cr',
'Mn',
'Fe',
'Co',
'Ni',
'Cu',
'Zn']
[27]:
abundance_scalings.GalacticConcordance().nitrogen(0.016)
[27]:
np.float64(-4.156584981878675)
These functions also include useful meta data:
[28]:
print(abundance_scalings.GalacticConcordance().ads)
print(abundance_scalings.GalacticConcordance().doi)
https://ui.adsabs.harvard.edu/abs/2017MNRAS.466.4403N/abstract
https://doi.org/10.1093/mnras/stw3235
Let’s dig a little deeper and make a plot of X/O vs. O/H for one of these scalings.
[29]:
scaling_study_name = "GalacticConcordance"
# create an array of metallicities equally space in log-space
log10metallicities = np.arange(-4.0, -1.5, 0.1)
metallicities = 10**log10metallicities
# the reference oxygen abundance for GalacticConcordance, i.e. [O/H]
reference_oxygen_abundance = -3.24
reference_metallicity = 0.015
# (O/H), assumed to scale linearly with metallicity
oxygen_abundance = reference_oxygen_abundance + np.log10(
metallicities / reference_metallicity
)
scaling_study = getattr(abundance_scalings, scaling_study_name)()
for element, element_name in zip(
scaling_study.available_elements, scaling_study.available_elements_names
):
scaling = getattr(scaling_study, element_name)
abundances = np.array(
[scaling(metallicity) for metallicity in metallicities]
)
# log10(N/O) = log10(N/H) - log10(O/H)
x_to_oxygen_ratios = abundances - oxygen_abundance
plt.plot(oxygen_abundance + 12.0, x_to_oxygen_ratios, label=element)
plt.plot(
[reference_oxygen_abundance + 12] * 2, [-6.0, -0.0], alpha=0.2, lw=2, c="k"
)
plt.ylabel(r"$\log_{10}(X/O)$")
plt.xlabel(r"$\log_{10}(O/H)+12$")
plt.legend()
plt.show()
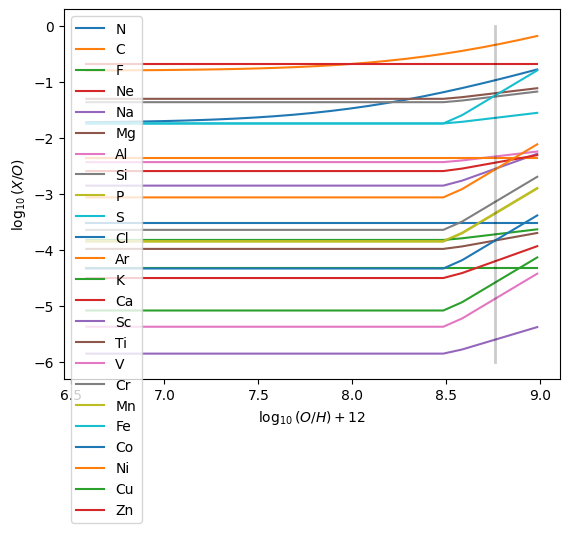
Plots¶
There are also a helper functions for plotting one or more abundance patterns, here we plot two abundance patterns with different alpha abundances:
[30]:
plot_multiple_abundance_patterns(
[a2, a3],
labels=[r"Z=0.01", r"Z=0.01; \alpha = 0.6"],
show=True,
ylim=[-7.0, -3.0],
)
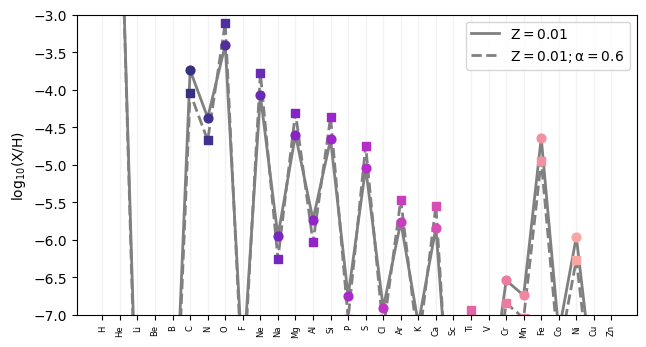
[30]:
(<Figure size 700x400 with 1 Axes>, <Axes: ylabel='$\\rm log_{10}(X/H)$'>)
We can plot the abundance pattern of each component:
[31]:
plot_abundance_pattern(
a7, show=True, ylim=[-7.0, -3.0], components=["total", "gas", "dust"]
)
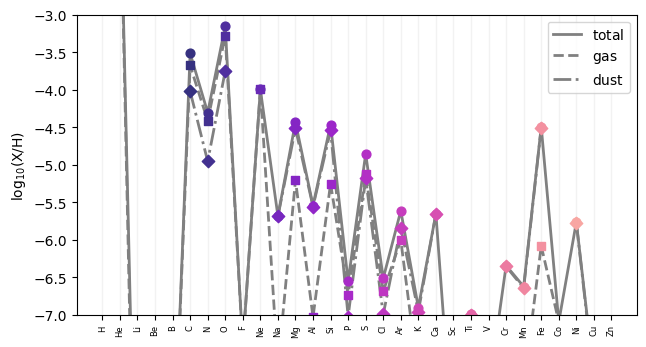
[31]:
(<Figure size 700x400 with 1 Axes>, <Axes: ylabel='$\\rm log_{10}(X/H)$'>)