synthesizer.components.component¶
A module containing generic component functionality.
This module contains the abstract base class for all components in the synthesizer. It defines the basic structure of a component and the methods that all components should have.
StellarComponents and BlackHoleComponents are children of this class and contain the specific functionality for stellar and black hole components respectively.
Classes
- class synthesizer.components.component.Component(component_type, **kwargs)[source]¶
The parent class for all components in the synthesizer.
This class contains the basic structure of a component and the methods that all components should have.
- component_type¶
The type of component, either “Stars” or “BlackHole”.
- Type:
str
- spectra¶
A dictionary to hold the stellar spectra.
- Type:
dict
- lines¶
A dictionary to hold the stellar emission lines.
- Type:
dict
- photo_lnu¶
A dictionary to hold the stellar photometry in luminosity units.
- Type:
dict
- photo_fnu¶
A dictionary to hold the stellar photometry in flux units.
- Type:
dict
- images_lnu¶
A dictionary to hold the images in luminosity units.
- Type:
dict
- images_fnu¶
A dictionary to hold the images in flux units
- Type:
dict
- clear_all_emissions()[source]¶
Clear all emissions from the component.
This clears all spectra, lines, and photometry.
- abstract generate_line(*args, **kwargs)[source]¶
Generate the rest frame line emission for the component.
- get_images_flux(resolution, fov, emission_model, img_type='smoothed', kernel=None, kernel_threshold=1, nthreads=1, limit_to=None, instrument=None)[source]¶
Make an ImageCollection from fluxes.
For Parametric components, images can only be smoothed. An exception will be raised if a histogram is requested.
For Particle components, images can either be a simple histogram (“hist”) or an image with particles smoothed over their SPH kernel.
Which images are produced is defined by the emission model. If any of the necessary photometry is missing for generating a particular image, an exception will be raised.
The limit_to argument can be used if only a specific image is desired.
Note that black holes will never be smoothed and only produce a histogram due to the point source nature of black holes.
All images that are created will be stored on the emitter (Stars or BlackHole/s) under the images_fnu attribute. The image collection at the root of the emission model will also be returned.
- Parameters:
resolution (Quantity, float) – The size of a pixel. (Ignoring any supersampling defined by psf_resample_factor)
fov – float The width of the image in image coordinates.
emission_model (EmissionModel) – The emission model to use to generate the images.
img_type – str The type of image to be made, either “hist” -> a histogram, or “smoothed” -> particles smoothed over a kernel for a particle galaxy. Otherwise, only smoothed is applicable.
stellar_photometry (string) – The stellar spectra key from which to extract photometry to use for the image.
blackhole_photometry (string) – The black hole spectra key from which to extract photometry to use for the image.
kernel (array-like, float) – The values from one of the kernels from the kernel_functions module. Only used for smoothed images.
kernel_threshold (float) – The kernel’s impact parameter threshold (by default 1).
nthreads (int) – The number of threads to use in the tree search. Default is 1.
instrument (Instrument) – The instrument to use to generate the images.
- Returns:
- array-like
A 2D array containing the image.
- Return type:
- get_images_luminosity(resolution, fov, emission_model, img_type='smoothed', kernel=None, kernel_threshold=1, nthreads=1, limit_to=None, instrument=None)[source]¶
Make an ImageCollection from component luminosities.
For Parametric components, images can only be smoothed. An exception will be raised if a histogram is requested.
For Particle components, images can either be a simple histogram (“hist”) or an image with particles smoothed over their SPH kernel.
Which images are produced is defined by the emission model. If any of the necessary photometry is missing for generating a particular image, an exception will be raised.
The limit_to argument can be used if only a specific image is desired.
Note that black holes will never be smoothed and only produce a histogram due to the point source nature of black holes.
All images that are created will be stored on the emitter (Stars or BlackHole/s) under the images_lnu attribute. The image collection at the root of the emission model will also be returned.
- Parameters:
resolution (Quantity, float) – The size of a pixel. (Ignoring any supersampling defined by psf_resample_factor)
fov – float The width of the image in image coordinates.
emission_model (EmissionModel) – The emission model to use to generate the images.
img_type – str The type of image to be made, either “hist” -> a histogram, or “smoothed” -> particles smoothed over a kernel for a particle galaxy. Otherwise, only smoothed is applicable.
stellar_photometry (string) – The stellar spectra key from which to extract photometry to use for the image.
blackhole_photometry (string) – The black hole spectra key from which to extract photometry to use for the image.
kernel (array-like, float) – The values from one of the kernels from the kernel_functions module. Only used for smoothed images.
kernel_threshold (float) – The kernel’s impact parameter threshold (by default 1).
nthreads (int) – The number of threads to use in the tree search. Default is 1.
instrument (Instrument) – The instrument to use to generate the images.
- Returns:
- array-like
A 2D array containing the image.
- Return type:
- get_lines(line_ids, emission_model, dust_curves=None, tau_v=None, fesc=0.0, mask=None, verbose=True, **kwargs)[source]¶
Generate stellar lines as described by the emission model.
- Parameters:
line_ids (list) – A list of line_ids. Doublets can be specified as a nested list or using a comma (e.g. ‘OIII4363,OIII4959’).
emission_model (EmissionModel) – The emission model to use.
dust_curves (dict) –
- An override to the emission model dust curves. Either:
None, indicating the dust_curves defined on the emission models should be used.
A single dust curve to apply to all emission models.
A dictionary of the form {<label>: <dust_curve instance>} to use a specific dust curve instance with particular properties.
tau_v (dict) –
- An override to the dust model optical depth. Either:
None, indicating the tau_v defined on the emission model should be used.
A float to use as the optical depth for all models.
A dictionary of the form {<label>: float(<tau_v>)} to use a specific optical depth with a particular model or {<label>: str(<attribute>)} to use an attribute of the component as the optical depth.
fesc (dict) –
- An override to the emission model escape fraction. Either:
None, indicating the fesc defined on the emission model should be used.
A float to use as the escape fraction for all models.
A dictionary of the form {<label>: float(<fesc>)} to use a specific escape fraction with a particular model or {<label>: str(<attribute>)} to use an attribute of the component as the escape fraction.
mask (dict) –
- An override to the emission model mask. Either:
None, indicating the mask defined on the emission model should be used.
A dictionary of the form {<label>: {“attr”: attr, “thresh”: thresh, “op”: op}} to add a specific mask to a particular model.
verbose (bool) – Are we talking?
kwargs (dict) – Any additional keyword arguments to pass to the generator function.
- Returns:
- LineCollection
A LineCollection object containing the lines defined by the root model.
- abstract get_mask(attr, thresh, op, mask=None)[source]¶
Return a mask based on the attribute and threshold.
- get_photo_fnu(filters, verbose=True, nthreads=1)[source]¶
Calculate flux photometry using a FilterCollection object.
- Parameters:
filters (object) – A FilterCollection object.
verbose (bool) – Are we talking?
nthreads (int) – The number of threads to use for the integration. If -1, all threads will be used.
- Returns:
- (dict)
A dictionary of fluxes in each filter in filters.
- get_photo_lnu(filters, verbose=True, nthreads=1)[source]¶
Calculate luminosity photometry using a FilterCollection object.
- Parameters:
filters (filters.FilterCollection) – A FilterCollection object.
verbose (bool) – Are we talking?
nthreads (int) – The number of threads to use for the integration. If -1, all threads will be used.
- Returns:
- photo_lnu (dict)
A dictionary of rest frame broadband luminosities.
- get_spectra(emission_model, dust_curves=None, tau_v=None, fesc=0.0, mask=None, verbose=True, **kwargs)[source]¶
Generate stellar spectra as described by the emission model.
- Parameters:
emission_model (EmissionModel) – The emission model to use.
dust_curves (dict) –
- An override to the emission model dust curves. Either:
None, indicating the dust_curves defined on the emission models should be used.
A single dust curve to apply to all emission models.
A dictionary of the form {<label>: <dust_curve instance>} to use a specific dust curve instance with particular properties.
tau_v (dict) –
- An override to the dust model optical depth. Either:
None, indicating the tau_v defined on the emission model should be used.
A float to use as the optical depth for all models.
A dictionary of the form {<label>: float(<tau_v>)} to use a specific optical depth with a particular model or {<label>: str(<attribute>)} to use an attribute of the component as the optical depth.
fesc (dict) –
- An override to the emission model escape fraction. Either:
None, indicating the fesc defined on the emission model should be used.
A float to use as the escape fraction for all models.
A dictionary of the form {<label>: float(<fesc>)} to use a specific escape fraction with a particular model or {<label>: str(<attribute>)} to use an attribute of the component as the escape fraction.
mask (dict) –
- An override to the emission model mask. Either:
None, indicating the mask defined on the emission model should be used.
A dictionary of the form {<label>: {“attr”: attr, “thresh”: thresh, “op”: op}} to add a specific mask to a particular model.
verbose (bool) – Are we talking?
kwargs (dict) – Any additional keyword arguments to pass to the generator function.
- Returns:
- dict
A dictionary of spectra which can be attached to the appropriate spectra attribute of the component (spectra/particle_spectra)
- property photo_fluxes¶
Get the photometric fluxes.
- Returns:
- dict
The photometry fluxes.
- property photo_luminosities¶
Get the photometric luminosities.
- Returns:
- dict
The photometry luminosities.
- plot_spectra(spectra_to_plot=None, show=False, ylimits=(), xlimits=(), figsize=(3.5, 5), **kwargs)[source]¶
Plot the spectra of the component.
Can either plot specific spectra (specified via spectra_to_plot) or all spectra on the child object.
- Parameters:
spectra_to_plot (string/list, string) –
- The specific spectra to plot.
If None all spectra are plotted.
If a list of strings each specifc spectra is plotted.
If a single string then only that spectra is plotted.
show (bool) – Flag for whether to show the plot or just return the figure and axes.
ylimits (tuple) – The limits to apply to the y axis. If not provided the limits will be calculated with the lower limit set to 1000 (100) times less than the peak of the spectrum for rest_frame (observed) spectra.
xlimits (tuple) – The limits to apply to the x axis. If not provided the optimal limits are found based on the ylimits.
figsize (tuple) – Tuple with size 2 defining the figure size.
kwargs (dict) – Arguments to the sed.plot_spectra method called from this wrapper.
- Returns:
- fig (matplotlib.pyplot.figure)
The matplotlib figure object for the plot.
- ax (matplotlib.axes)
The matplotlib axes object containing the plotted data.
Examples using synthesizer.components.component.Component
¶
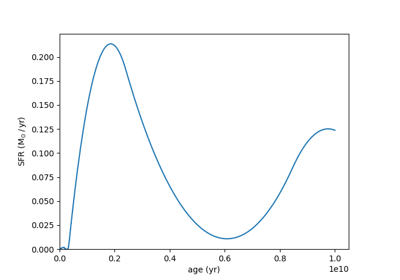
Demonstrate the dense basis approach for describing the SFZH
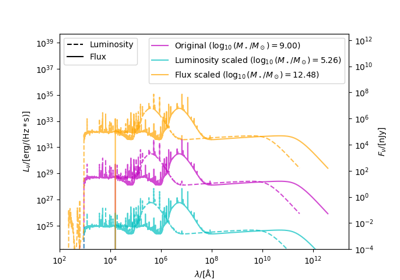
An example showing how to scale a galaxy’s mass by luminosity/flux.
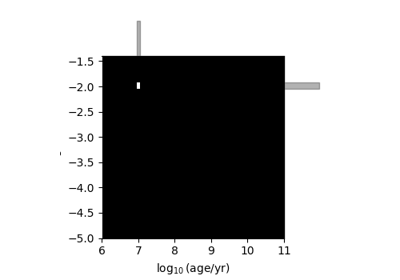
Compare Single star particle to instantaneous SFZH