synthesizer.base_galaxy¶
A module for common functionality in Parametric and Particle Galaxies
The class described in this module should never be directly instatiated. It only contains common attributes and methods to reduce boilerplate.
Classes
- class synthesizer.base_galaxy.BaseGalaxy(stars, gas, black_holes, redshift, centre, **kwargs)[source]¶
The base galaxy class.
This should never be directly instantiated. It instead contains the common functionality and attributes needed for parametric and particle galaxies.
- spectra¶
The dictionary containing a Galaxy’s spectra. Each entry is an Sed object. This dictionary only contains combined spectra from All components that make up the Galaxy (Stars, Gas, BlackHoles).
- Type:
dict, Sed
- stars¶
The Stars object holding information about the stellar population.
- Type:
particle.Stars/parametric.Stars
- gas¶
The Gas object holding information about the gas distribution.
- Type:
particle.Gas/parametric.Gas
- black_holes¶
The BlackHole/s object holding information about the black hole/s.
- Type:
particle.BlackHoles/parametric.BlackHole
- clear_all_emissions()[source]¶
Clear all spectra, lines and photometry.
This method is a quick helper to clear all spectra, lines, and photometry from the galaxy object and its components. This will cover both integrated and per particle emission.
- clear_all_lines()[source]¶
Clear all lines.
This method is a quick helper to clear all lines from the galaxy object and its components. This will cover both integrated and per particle lines if present.
- clear_all_photometry()[source]¶
Clear all photometry.
This method is a quick helper to clear all photometry from the galaxy object and its components. This will cover both integrated and per particle photometry if present.
- clear_all_spectra()[source]¶
Clear all spectra.
This method is a quick helper to clear all spectra from the galaxy object and its components. This will cover both integrated and per particle spectra if present.
- get_equivalent_width(feature, blue, red, spectra_to_plot=None)[source]¶
Get all equivalent widths associated with a sed object
- Parameters:
index (float) – the index to be used in the computation of equivalent width.
spectra_to_plot (float array) – An empty list of spectra to be populated.
- Returns:
equivalent_width – The calculated equivalent width at the current index.
- Return type:
float
- get_images_flux(resolution, fov, emission_model, img_type='smoothed', kernel=None, kernel_threshold=1, nthreads=1, limit_to=None, instrument=None)[source]¶
Make an ImageCollection from fluxes.
For Parametric Galaxy objects, images can only be smoothed. An exception will be raised if a histogram is requested.
For Particle Galaxy objects, images can either be a simple histogram (“hist”) or an image with particles smoothed over their SPH kernel.
Which images are produced is defined by the emission model. If any of the necessary photometry is missing for generating a particular image, an exception will be raised.
The limit_to argument can be used if only a specific image is desired.
Note that black holes will never be smoothed and only produce a histogram due to the point source nature of black holes.
All images that are created will be stored on the emitter (Stars, BlackHole/s, or galaxy) under the images_fnu attribute. The image collection at the root of the emission model will also be returned.
- Parameters:
resolution (Quantity, float) – The size of a pixel. (Ignoring any supersampling defined by psf_resample_factor)
fov – float The width of the image in image coordinates.
emission_model (EmissionModel) – The emission model to use to generate the images.
img_type – str The type of image to be made, either “hist” -> a histogram, or “smoothed” -> particles smoothed over a kernel for a particle galaxy. Otherwise, only smoothed is applicable.
kernel (array-like, float) – The values from one of the kernels from the kernel_functions module. Only used for smoothed images.
kernel_threshold (float) – The kernel’s impact parameter threshold (by default 1).
nthreads (int) – The number of threads to use in the tree search. Default is 1.
limit_to (str) – Optionally pass a single model label to limit image generation to only that model.
instrument (Instrument) – The instrument to use for the image. This can be None but if not it will be used to limit the included filters and label the images by instrument.
- Returns:
- array-like
A 2D array containing the image.
- Return type:
- get_images_luminosity(resolution, fov, emission_model, img_type='smoothed', kernel=None, kernel_threshold=1, nthreads=1, limit_to=None, instrument=None)[source]¶
Make an ImageCollection from luminosities.
For Parametric Galaxy objects, images can only be smoothed. An exception will be raised if a histogram is requested.
For Particle Galaxy objects, images can either be a simple histogram (“hist”) or an image with particles smoothed over their SPH kernel.
Which images are produced is defined by the emission model. If any of the necessary photometry is missing for generating a particular image, an exception will be raised.
The limit_to argument can be used if only a specific image is desired.
Note that black holes will never be smoothed and only produce a histogram due to the point source nature of black holes.
All images that are created will be stored on the emitter (Stars, BlackHole/s, or galaxy) under the images_lnu attribute. The image collection at the root of the emission model will also be returned.
- Parameters:
resolution (Quantity, float) – The size of a pixel. (Ignoring any supersampling defined by psf_resample_factor)
fov – float The width of the image in image coordinates.
emission_model (EmissionModel) – The emission model to use to generate the images.
img_type – str The type of image to be made, either “hist” -> a histogram, or “smoothed” -> particles smoothed over a kernel for a particle galaxy. Otherwise, only smoothed is applicable.
stellar_photometry (string) – The stellar spectra key from which to extract photometry to use for the image.
blackhole_photometry (string) – The black hole spectra key from which to extract photometry to use for the image.
kernel (array-like, float) – The values from one of the kernels from the kernel_functions module. Only used for smoothed images.
kernel_threshold (float) – The kernel’s impact parameter threshold (by default 1).
nthreads (int) – The number of threads to use in the tree search. Default is 1.
limit_to (str) – Optionally pass a single model label to limit image generation to only that model.
instrument (Instrument) – The instrument to use for the image. This can be None but if not it will be used to limit the included filters and label the images by instrument.
- Returns:
- array-like
A 2D array containing the image.
- Return type:
- get_lines(line_ids, emission_model, dust_curves=None, tau_v=None, fesc=0.0, covering_fraction=None, mask=None, verbose=True, **kwargs)[source]¶
Generate lines as described by the emission model.
- Parameters:
line_ids (list) – A list of line ids to include in the spectra.
emission_model (EmissionModel) – The emission model to use.
dust_curves (dict) –
- An override to the emission model dust curves. Either:
None, indicating the dust_curves defined on the emission models should be used.
A single dust curve to apply to all emission models.
A dictionary of the form {<label>: <dust_curve instance>} to use a specific dust curve instance with particular properties.
tau_v (dict) –
- An override to the dust model optical depth. Either:
None, indicating the tau_v defined on the emission model should be used.
A float to use as the optical depth for all models.
A dictionary of the form {<label>: float(<tau_v>)} to use a specific optical depth with a particular model or {<label>: str(<attribute>)} to use an attribute of the component as the optical depth.
fesc (dict) –
- An override to the emission model escape fraction. Either:
None, indicating the fesc defined on the emission model should be used.
A float to use as the escape fraction for all models.
A dictionary of the form {<label>: float(<fesc>)} to use a specific escape fraction with a particular model or {<label>: str(<attribute>)} to use an attribute of the component as the escape fraction.
mask (dict) –
- An override to the emission model mask. Either:
None, indicating the mask defined on the emission model should be used.
A dictionary of the form {<label>: {“attr”: attr, “thresh”: thresh, “op”: op}} to add a specific mask to a particular model.
verbose (bool) – Are we talking?
kwargs (dict) – Any additional keyword arguments to pass to the generator function.
- Returns:
- dict
The combined lines for the galaxy.
- get_observed_spectra(cosmo, igm=<class 'synthesizer.emission_models.attenuation.igm.Inoue14'>)[source]¶
Calculate the observed spectra for all Sed objects within this galaxy.
This will run Sed.get_fnu(…) and populate Sed.fnu (and sed.obslam and sed.obsnu) for all spectra in: - Galaxy.spectra - Galaxy.stars.spectra - Galaxy.gas.spectra (WIP) - Galaxy.black_holes.spectra
And in the case of particle galaxies - Galaxy.stars.particle_spectra - Galaxy.gas.particle_spectra (WIP) - Galaxy.black_holes.particle_spectra
- Parameters:
cosmo (astropy.cosmology.Cosmology) – The cosmology object containing the cosmological model used to calculate the luminosity distance.
igm (igm) – The object describing the intergalactic medium (defaults to Inoue14).
- Raises:
MissingAttribute – If a galaxy has no redshift we can’t get the observed spectra.
- get_photo_fnu(filters, verbose=True, nthreads=1)[source]¶
Calculate flux photometry using a FilterCollection object.
Photometry is calculated in spectral flux density units.
- Parameters:
filters (object) – A FilterCollection object.
verbose (bool) – Are we talking?
nthreads (int) – The number of threads to use for the integration. If -1, all threads will be used.
- Returns:
- PhotometryCollection
A PhotometryCollection object containing the flux photometry in each filter in filters.
- get_photo_lnu(filters, verbose=True, nthreads=1)[source]¶
Calculate luminosity photometry using a FilterCollection object.
Photometry is calculated in spectral luminosity density units.
- Parameters:
filters (filters.FilterCollection) – A FilterCollection object.
verbose (bool) – Are we talking?
nthreads (int) – The number of threads to use for the integration. If -1, all threads will be used.
- Returns:
- PhotometryCollection
A PhotometryCollection object containing the luminosity photometry in each filter in filters.
- get_spectra(emission_model, dust_curves=None, tau_v=None, fesc=0.0, covering_fraction=None, mask=None, verbose=True, **kwargs)[source]¶
Generate spectra as described by the emission model.
- Parameters:
emission_model (EmissionModel) – The emission model to use.
dust_curves (dict) –
- An override to the emission model dust curves. Either:
None, indicating the dust_curves defined on the emission models should be used.
A single dust curve to apply to all emission models.
A dictionary of the form {<label>: <dust_curve instance>} to use a specific dust curve instance with particular properties.
tau_v (dict) –
- An override to the dust model optical depth. Either:
None, indicating the tau_v defined on the emission model should be used.
A float to use as the optical depth for all models.
A dictionary of the form {<label>: float(<tau_v>)} to use a specific optical depth with a particular model or {<label>: str(<attribute>)} to use an attribute of the component as the optical depth.
fesc (dict) –
- An override to the emission model escape fraction. Either:
None, indicating the fesc defined on the emission model should be used.
A float to use as the escape fraction for all models.
A dictionary of the form {<label>: float(<fesc>)} to use a specific escape fraction with a particular model or {<label>: str(<attribute>)} to use an attribute of the component as the escape fraction.
mask (dict) –
- An override to the emission model mask. Either:
None, indicating the mask defined on the emission model should be used.
A dictionary of the form {<label>: {“attr”: attr, “thresh”: thresh, “op”: op}} to add a specific mask to a particular model.
verbose (bool) – Are we talking?
kwargs (dict) – Any additional keyword arguments to pass to the generator function.
- Returns:
- dict
The combined spectra for the galaxy.
- get_spectra_combined()[source]¶
Combine all common component spectra from components onto the galaxy.
- e.g.:
intrinsc = stellar_intrinsic + black_hole_intrinsic.
For any combined spectra all components with a valid spectra will be combined and stored in Galaxy.spectra under the same key, but only if there are instances of that spectra key on more than 1 component.
- Possible combined spectra are:
“total”
“intrinsic”
“emergent”
Note that this process is only applicable to integrated spectra.
- property photo_fluxes¶
Get the photometry fluxes.
- Returns:
- dict
The photometry fluxes.
- property photo_luminosities¶
Get the photometry luminosities.
- Returns:
- dict
The photometry luminosities.
- plot_observed_spectra(combined_spectra=True, stellar_spectra=False, gas_spectra=False, black_hole_spectra=False, show=False, ylimits=(), xlimits=(), figsize=(3.5, 5), filters=None, quantity_to_plot='fnu')[source]¶
Plots either specific observed spectra (specified via combined_spectra, stellar_spectra, gas_spectra, and/or black_hole_spectra) or all spectra for any of the spectra arguments that are True. If any are false that component is ignored.
- Parameters:
combined_spectra (bool/list, string/string) –
- The specific combined galaxy spectra to plot. (e.g “total”)
If True all spectra are plotted.
If a list of strings each specifc spectra is plotted.
If a single string then only that spectra is plotted.
stellar_spectra (bool/list, string/string) –
- The specific stellar spectra to plot. (e.g. “incident”)
If True all spectra are plotted.
If a list of strings each specifc spectra is plotted.
If a single string then only that spectra is plotted.
gas_spectra (bool/list, string/string) –
- The specific gas spectra to plot. (e.g. “total”)
If True all spectra are plotted.
If a list of strings each specifc spectra is plotted.
If a single string then only that spectra is plotted.
black_hole_spectra (bool/list, string/string) –
- The specific black hole spectra to plot. (e.g “blr”)
If True all spectra are plotted.
If a list of strings each specifc spectra is plotted.
If a single string then only that spectra is plotted.
show (bool) – Flag for whether to show the plot or just return the figure and axes.
ylimits (tuple) – The limits to apply to the y axis. If not provided the limits will be calculated with the lower limit set to 1000 (100) times less than the peak of the spectrum for rest_frame (observed) spectra.
xlimits (tuple) – The limits to apply to the x axis. If not provided the optimal limits are found based on the ylimits.
figsize (tuple) – Tuple with size 2 defining the figure size.
filters (FilterCollection) – If given then the photometry is computed and both the photometry and filter curves are plotted
quantity_to_plot (string) – The sed property to plot. Can be “lnu”, “luminosity” or “llam” for rest frame spectra or “fnu”, “flam” or “flux” for observed spectra. Defaults to “lnu”.
- Returns:
- fig (matplotlib.pyplot.figure)
The matplotlib figure object for the plot.
- ax (matplotlib.axes)
The matplotlib axes object containing the plotted data.
- plot_spectra(combined_spectra=True, stellar_spectra=False, gas_spectra=False, black_hole_spectra=False, show=False, ylimits=(), xlimits=(), figsize=(3.5, 5), quantity_to_plot='lnu')[source]¶
Plots either specific observed spectra (specified via combined_spectra, stellar_spectra, gas_spectra, and/or black_hole_spectra) or all spectra for any of the spectra arguments that are True. If any are false that component is ignored.
- Parameters:
combined_spectra (bool/list, string/string) –
- The specific combined galaxy spectra to plot. (e.g “total”)
If True all spectra are plotted.
If a list of strings each specifc spectra is plotted.
If a single string then only that spectra is plotted.
stellar_spectra (bool/list, string/string) –
- The specific stellar spectra to plot. (e.g. “incident”)
If True all spectra are plotted.
If a list of strings each specifc spectra is plotted.
If a single string then only that spectra is plotted.
gas_spectra (bool/list, string/string) –
- The specific gas spectra to plot. (e.g. “total”)
If True all spectra are plotted.
If a list of strings each specifc spectra is plotted.
If a single string then only that spectra is plotted.
black_hole_spectra (bool/list, string/string) –
- The specific black hole spectra to plot. (e.g “blr”)
If True all spectra are plotted.
If a list of strings each specifc spectra is plotted.
If a single string then only that spectra is plotted.
show (bool) – Flag for whether to show the plot or just return the figure and axes.
ylimits (tuple) – The limits to apply to the y axis. If not provided the limits will be calculated with the lower limit set to 1000 (100) times less than the peak of the spectrum for rest_frame (observed) spectra.
xlimits (tuple) – The limits to apply to the x axis. If not provided the optimal limits are found based on the ylimits.
figsize (tuple) – Tuple with size 2 defining the figure size.
quantity_to_plot (string) – The sed property to plot. Can be “lnu”, “luminosity” or “llam” for rest frame spectra or “fnu”, “flam” or “flux” for observed spectra. Defaults to “lnu”.
- Returns:
- fig (matplotlib.pyplot.figure)
The matplotlib figure object for the plot.
- ax (matplotlib.axes)
The matplotlib axes object containing the plotted data.
Examples using synthesizer.base_galaxy.BaseGalaxy
¶
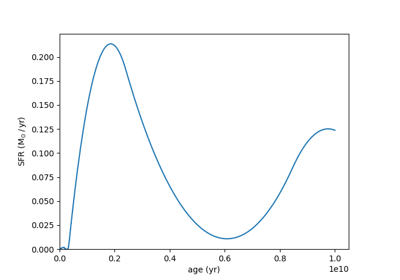
Demonstrate the dense basis approach for describing the SFZH