synthesizer.components.stellar¶
A module defining general functionality for particle and parametric stars.
This should never be directly instantiated. Instead it is the parent class for particle.Stars and parametric.Stars and contains attributes and methods common between them. StarsComponent is a child class of Component.
Classes
- class synthesizer.components.stellar.StarsComponent(ages, metallicities, _star_type, **kwargs)[source]¶
The parent class for stellar components of a galaxy.
This class contains the attributes and spectra creation methods which are common to both parametric and particle stellar components.
This should never be instantiated directly.
- property log10ages¶
Return stellar particle ages in log (base 10).
- Returns:
- log10ages (array)
log10 stellar ages
- property log10metallicities¶
Return stellar particle metallicities in log (base 10).
- Returns:
- log10metallicities (array)
log10 stellar metallicities
Examples using synthesizer.components.stellar.StarsComponent
¶
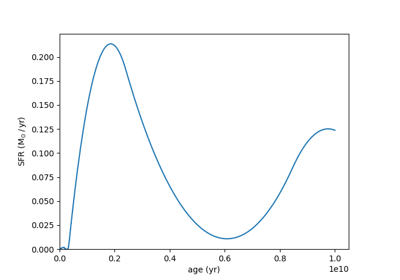
Demonstrate the dense basis approach for describing the SFZH
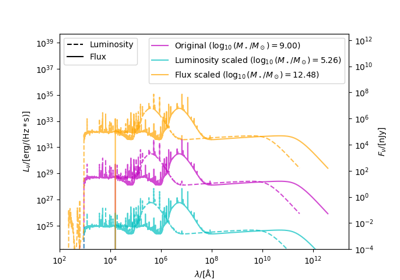
An example showing how to scale a galaxy’s mass by luminosity/flux.
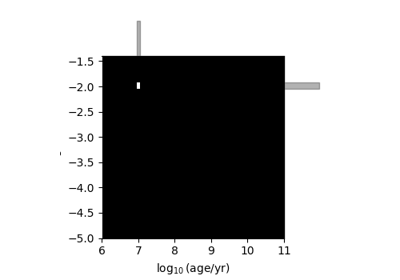
Compare Single star particle to instantaneous SFZH