Note
Go to the end to download the full example code.
Create sampled SED¶
this example generates a sample of star particles from a 2D SFZH. In this case it is generated from a parametric star formation history with constant star formation.
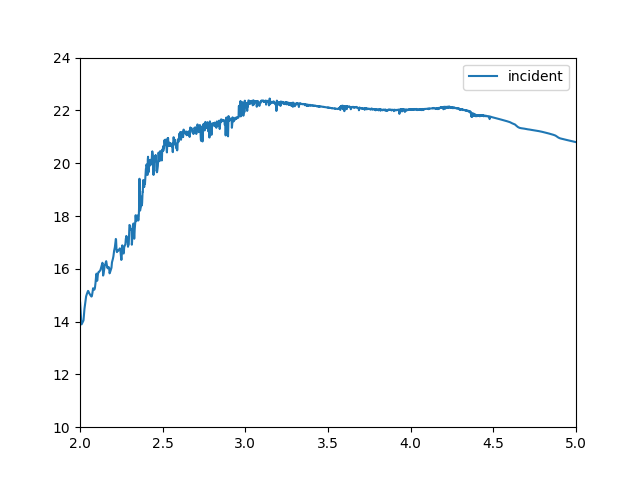
+-------------------------------------------------------------------------------------------------------+
| STARS |
+--------------------------+----------------------------------------------------------------------------+
| Attribute | Value |
+--------------------------+----------------------------------------------------------------------------+
| component_type | 'Stars' |
+--------------------------+----------------------------------------------------------------------------+
| sf_hist_func | <synthesizer.parametric.sf_hist.Constant object at 0x7f1af2008b50> |
+--------------------------+----------------------------------------------------------------------------+
| metal_dist_func | <synthesizer.parametric.metal_dist.DeltaConstant object at 0x7f1af200a5c0> |
+--------------------------+----------------------------------------------------------------------------+
| metallicity_grid_type | 'log10Z' |
+--------------------------+----------------------------------------------------------------------------+
| log10ages_lims | [6.0, 10.399999999999984] |
+--------------------------+----------------------------------------------------------------------------+
| metallicities_lims | [1e-05, 0.025118864315095104] |
+--------------------------+----------------------------------------------------------------------------+
| log10metallicities_lims | [-5.0, -1.600000000000012] |
+--------------------------+----------------------------------------------------------------------------+
| ages (45,) | 1.00e+06 yr -> 2.51e+10 yr (Mean: 2.71e+09 yr) |
+--------------------------+----------------------------------------------------------------------------+
| metallicities (35,) | 1.00e-05 -> 2.51e-02 (Mean: 3.49e-03) |
+--------------------------+----------------------------------------------------------------------------+
| sf_hist (45,) | 0.00e+00 -> 1.85e+08 (Mean: 2.22e+07) |
+--------------------------+----------------------------------------------------------------------------+
| metal_dist (35,) | 0.00e+00 -> 1.00e+09 (Mean: 2.86e+07) |
+--------------------------+----------------------------------------------------------------------------+
| initial_mass | 999999999.9999999 Msun |
+--------------------------+----------------------------------------------------------------------------+
| sfzh (45, 35) | 0.00e+00 -> 1.85e+08 (Mean: 6.35e+05) |
+--------------------------+----------------------------------------------------------------------------+
| log10ages (45,) | 6.00e+00 -> 1.04e+01 (Mean: 8.20e+00) |
+--------------------------+----------------------------------------------------------------------------+
| log10metallicities (35,) | -5.00e+00 -> -1.60e+00 (Mean: -3.30e+00) |
+--------------------------+----------------------------------------------------------------------------+
import matplotlib.pyplot as plt
import numpy as np
from unyt import Msun, Myr
from synthesizer.emission_models import IncidentEmission
from synthesizer.grid import Grid
from synthesizer.parametric import SFH, ZDist
from synthesizer.parametric import Stars as ParametricStars
from synthesizer.particle.galaxy import Galaxy
from synthesizer.particle.stars import sample_sfzh
# Define the grid
grid_name = "test_grid"
grid_dir = "../../tests/test_grid/"
grid = Grid(grid_name, grid_dir=grid_dir)
# Define the emission model
model = IncidentEmission(grid)
# define the grid (normally this would be defined by an SPS grid)
log10ages = np.arange(6.0, 10.5, 0.1)
metallicities = 10 ** np.arange(-5.0, -1.5, 0.1)
# define the parameters of the star formation and metal enrichment histories
Z_p = {"metallicity": 0.01}
metal_dist = ZDist.DeltaConstant(**Z_p)
sfh_p = {"duration": 100 * Myr}
sfh = SFH.Constant(**sfh_p) # constant star formation
sfzh = ParametricStars(
log10ages,
metallicities,
sf_hist=sfh,
metal_dist=metal_dist,
initial_mass=10**9 * Msun,
)
print(sfzh)
# sfzh.plot()
# --- create stars object
N = 100 # number of particles for sampling
stars = sample_sfzh(sfzh.sfzh, sfzh.log10ages, sfzh.log10metallicities, N)
# --- create galaxy object
galaxy = Galaxy(stars=stars)
""" this generates stellar and intrinsic spectra
galaxy.generate_intrinsic_spectra(grid, fesc=0.0)
calculate only integrated SEDs """
galaxy.stars.get_spectra(model)
for sed_type, sed in galaxy.stars.spectra.items():
plt.plot(np.log10(sed.lam), np.log10(sed.lnu), label=sed_type)
plt.legend()
plt.xlim([2, 5])
plt.ylim([10, 24])
plt.show()
Total running time of the script: (0 minutes 0.431 seconds)