Generate composite galaxy¶
Finall, in this example we’re going to demonstrate how to make a composite galaxy, including with imaging. For more information on defining parametric morphology see the Imaging examples.
[1]:
import matplotlib.pyplot as plt
import numpy as np
from synthesizer.filters import UVJ
from synthesizer.grid import Grid
from synthesizer.parametric import SFH, Stars, ZDist
from synthesizer.parametric.galaxy import Galaxy
from synthesizer.parametric.morphology import Sersic2D
from unyt import Myr, kpc
Let’s begin by defining the geometry of the images:
[2]:
# Define geometry of the images
resolution = 0.1 * kpc # resolution in kpc
npix = 50
fov = resolution * npix
And initialising the Grid
object:
[3]:
grid_dir = "../../../tests/test_grid"
grid_name = "test_grid"
grid = Grid(grid_name, grid_dir=grid_dir, new_lam=np.logspace(3, 4.2, 1000))
And initialising a FilterCollection
, in this case the rest-frame UVJ filters.
[4]:
filters = UVJ(new_lam=grid.lam)
Disk¶
Let’s now build the disk component of our composite galaxy.
Starting by defining the morphology:
[5]:
morph = Sersic2D(
r_eff=1.0 * kpc, sersic_index=1.0, ellipticity=0.5, theta=35.0
)
Define the parameters of the star formation and metal enrichment histories
[6]:
sfh_p = {"duration": 10 * Myr}
Z_p = {
"log10metallicity": -2.0
} # can also use linear metallicity e.g. {'Z': 0.01}
stellar_mass = 10**9.5
Define the functional form of the star formation and metal enrichment histories
[7]:
sfh = SFH.Constant(**sfh_p) # constant star formation
metal_dist = ZDist.DeltaConstant(**Z_p) # constant metallicity
Get the 2D star formation and metal enrichment history for the given SPS grid. This is (age, Z).
[8]:
stars = Stars(
grid.log10age,
grid.metallicity,
sf_hist=sfh,
metal_dist=metal_dist,
initial_mass=stellar_mass,
morphology=morph,
)
Initialise the Galaxy object, make an image and plot it. In this case, we can make a colour image using our UVJ filters:
[9]:
# Initialise Galaxy object
disk = Galaxy(stars=stars)
# Generate stellar spectra
disk.stars.get_spectra_incident(grid)
# Get photometry
disk.stars.spectra["incident"].get_photo_luminosities(filters)
# Make images
disk_img = disk.get_images_luminosity(
resolution=resolution,
stellar_photometry="incident",
fov=fov,
)
print(disk)
# Make and plot an rgb image
disk_img.make_rgb_image(rgb_filters={"R": "J", "G": "V", "B": "U"})
fig, ax, _ = disk_img.plot_rgb_image(show=True)
-------------------------------------------------------------------------------
SUMMARY OF PARAMETRIC GALAXY
⠀⠀⠀⠀⠀⠀⠀⠀⠀⠀⢀⣀⣀⡀⠒⠒⠦⣄⡀⠀⠀⠀⠀⠀⠀⠀
⠀⠀⠀⠀⠀⢀⣤⣶⡾⠿⠿⠿⠿⣿⣿⣶⣦⣄⠙⠷⣤⡀⠀⠀⠀⠀
⠀⠀⠀⣠⡾⠛⠉⠀⠀⠀⠀⠀⠀⠀⠈⠙⠻⣿⣷⣄⠘⢿⡄⠀⠀⠀
⠀⢀⡾⠋⠀⠀⠀⠀⠀⠀⠀⠀⠐⠂⠠⢄⡀⠈⢿⣿⣧⠈⢿⡄⠀⠀
⢀⠏⠀⠀⠀⢀⠄⣀⣴⣾⠿⠛⠛⠛⠷⣦⡙⢦⠀⢻⣿⡆⠘⡇⠀⠀
⠀⠀⠀+-+-+-+-+-+-+-+-+-+-+-+⡇⠀⠀
⠀⠀⠀|S|Y|N|T|H|E|S|I|Z|E|R|⠃⠀⠀
⠀⠀⢰+-+-+-+-+-+-+-+-+-+-+-+⠀⠀⠀
⠀⠀⢸⡇⠸⣿⣷⠀⢳⡈⢿⣦⣀⣀⣀⣠⣴⣾⠟⠁⠀⠀⠀⠀⢀⡎
⠀⠀⠘⣷⠀⢻⣿⣧⠀⠙⠢⠌⢉⣛⠛⠋⠉⠀⠀⠀⠀⠀⠀⣠⠎⠀
⠀⠀⠀⠹⣧⡀⠻⣿⣷⣄⡀⠀⠀⠀⠀⠀⠀⠀⠀⠀⢀⣠⡾⠃⠀⠀
⠀⠀⠀⠀⠈⠻⣤⡈⠻⢿⣿⣷⣦⣤⣤⣤⣤⣤⣴⡾⠛⠉⠀⠀⠀⠀
⠀⠀⠀⠀⠀⠀⠈⠙⠶⢤⣈⣉⠛⠛⠛⠛⠋⠉⠀⠀⠀⠀⠀⠀⠀⠀
⠀⠀⠀⠀⠀⠀⠀⠀⠀⠀⠀⠀⠉⠉⠉⠁⠀⠀⠀⠀⠀⠀⠀⠀⠀⠀
<class 'synthesizer.parametric.galaxy.Galaxy'>
log10(stellar mass formed/Msol): 9.5
available SEDs:
Stellar: [incident]
Black Holes: []
Combined: []
available lines: []
available images: []
-------------------------------------------------------------------------------
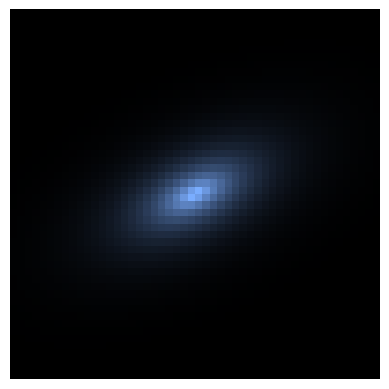
Bulge¶
Lets do the same but make a Bulge this time to combine.
[10]:
# Define bulge morphology
morph = Sersic2D(r_eff=0.5 * kpc, sersic_index=4.0)
# Define the parameters of the star formation and metal enrichment histories
stellar_mass = 10**8.5
stars = Stars(
grid.log10age,
grid.metallicity,
sf_hist=10.0 * Myr,
metal_dist=0.01,
morphology=morph,
initial_mass=stellar_mass,
)
# Get galaxy object
bulge = Galaxy(stars=stars)
# Get spectra
bulge.stars.get_spectra_incident(grid)
# Get photometry
bulge.stars.spectra["incident"].get_photo_luminosities(filters)
# make images
bulge_img = bulge.get_images_luminosity(
resolution=resolution,
stellar_photometry="incident",
fov=fov,
)
# Make and plot an rgb image
bulge_img.make_rgb_image(rgb_filters={"R": "J", "G": "V", "B": "U"})
fig, ax, _ = bulge_img.plot_rgb_image(show=True)
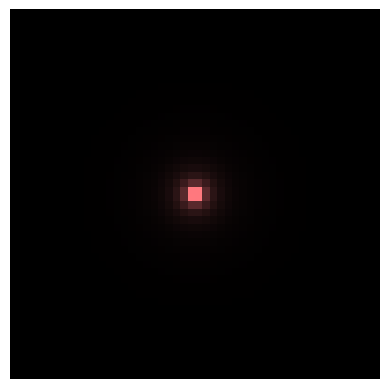
Total¶
Finally we can combine the disk and bulge together to make a composite galaxy.
[11]:
# Combine galaxy objects
combined = disk + bulge
print(combined)
# Combine images
total = disk_img + bulge_img
# Make and plot an rgb image
total.make_rgb_image(rgb_filters={"R": "J", "G": "V", "B": "U"})
fig, ax, _ = total.plot_rgb_image(show=True)
-------------------------------------------------------------------------------
SUMMARY OF PARAMETRIC GALAXY
⠀⠀⠀⠀⠀⠀⠀⠀⠀⠀⢀⣀⣀⡀⠒⠒⠦⣄⡀⠀⠀⠀⠀⠀⠀⠀
⠀⠀⠀⠀⠀⢀⣤⣶⡾⠿⠿⠿⠿⣿⣿⣶⣦⣄⠙⠷⣤⡀⠀⠀⠀⠀
⠀⠀⠀⣠⡾⠛⠉⠀⠀⠀⠀⠀⠀⠀⠈⠙⠻⣿⣷⣄⠘⢿⡄⠀⠀⠀
⠀⢀⡾⠋⠀⠀⠀⠀⠀⠀⠀⠀⠐⠂⠠⢄⡀⠈⢿⣿⣧⠈⢿⡄⠀⠀
⢀⠏⠀⠀⠀⢀⠄⣀⣴⣾⠿⠛⠛⠛⠷⣦⡙⢦⠀⢻⣿⡆⠘⡇⠀⠀
⠀⠀⠀+-+-+-+-+-+-+-+-+-+-+-+⡇⠀⠀
⠀⠀⠀|S|Y|N|T|H|E|S|I|Z|E|R|⠃⠀⠀
⠀⠀⢰+-+-+-+-+-+-+-+-+-+-+-+⠀⠀⠀
⠀⠀⢸⡇⠸⣿⣷⠀⢳⡈⢿⣦⣀⣀⣀⣠⣴⣾⠟⠁⠀⠀⠀⠀⢀⡎
⠀⠀⠘⣷⠀⢻⣿⣧⠀⠙⠢⠌⢉⣛⠛⠋⠉⠀⠀⠀⠀⠀⠀⣠⠎⠀
⠀⠀⠀⠹⣧⡀⠻⣿⣷⣄⡀⠀⠀⠀⠀⠀⠀⠀⠀⠀⢀⣠⡾⠃⠀⠀
⠀⠀⠀⠀⠈⠻⣤⡈⠻⢿⣿⣷⣦⣤⣤⣤⣤⣤⣴⡾⠛⠉⠀⠀⠀⠀
⠀⠀⠀⠀⠀⠀⠈⠙⠶⢤⣈⣉⠛⠛⠛⠛⠋⠉⠀⠀⠀⠀⠀⠀⠀⠀
⠀⠀⠀⠀⠀⠀⠀⠀⠀⠀⠀⠀⠉⠉⠉⠁⠀⠀⠀⠀⠀⠀⠀⠀⠀⠀
<class 'synthesizer.parametric.galaxy.Galaxy'>
log10(stellar mass formed/Msol): 9.541392685158225
available SEDs:
Stellar: [incident]
Black Holes: []
Combined: []
available lines: []
available images: []
-------------------------------------------------------------------------------
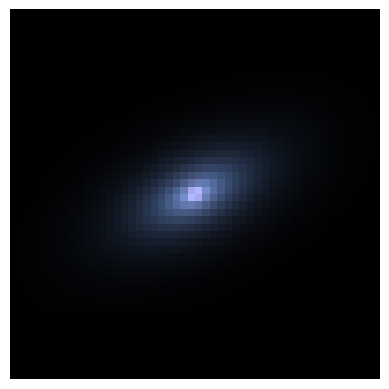
And plot the spectra for good measure…
[12]:
sed = disk.stars.spectra["incident"]
plt.plot(
np.log10(sed.lam), np.log10(sed.lnu), lw=1, alpha=0.8, c="b", label="disk"
)
sed = bulge.stars.spectra["incident"]
plt.plot(
np.log10(sed.lam), np.log10(sed.lnu), lw=1, alpha=0.8, c="r", label="bulge"
)
sed = combined.stars.spectra["incident"]
plt.plot(
np.log10(sed.lam),
np.log10(sed.lnu),
lw=2,
alpha=0.8,
c="k",
label="combined",
)
plt.xlim(3.0, 4.3)
plt.legend(fontsize=8, labelspacing=0.0)
plt.xlabel(r"$\rm log_{10}(\lambda_{obs}/\AA)$")
plt.ylabel(r"$\rm log_{10}(f_{\nu}/nJy)$")
plt.show()
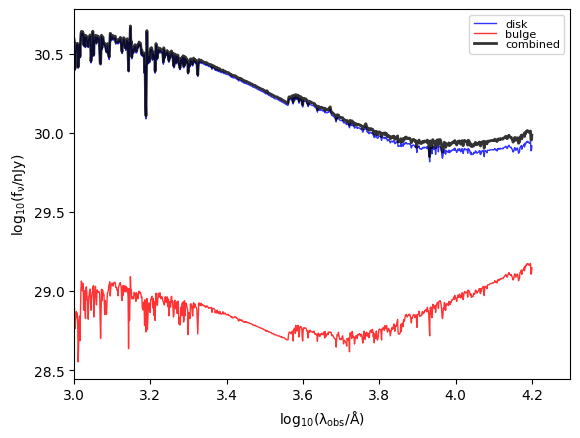